 |
 |
|
 |
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
More SSLT fun, and maybe I've got this all wrong, but I'm following Jaime's
wise ignorancia philosophy and this is working for me right now...
Please pardon the code here, but I think it's nice posted all in one spot like
this:
//==============================================================================
// Persistence of Vision Ray Tracer Scene Description File
//-----------------------------------------------------------------------------
// File : sslt_spheres.pov
// Version : 3.7
// Description : Playing with SSLT stuff
// Date : 09/21/2010
// Author : Robert W. McGregor, rob### [at] mcgregorFineArt com
//==============================================================================
#version 3.7; // beta 39
default { pigment { color rgb 0.75 } finish { ambient 0 diffuse 0.5 }}
#include "colors.inc"
#include "math.inc"
#include "rand.inc"
//------------------------------------------------------------------------------
// Globals and radiosity settings
//------------------------------------------------------------------------------
#declare R = seed(1234);
#declare Soft_Shadows = 1;
#declare Use_Radiosity = 1;
#declare SCALE = 100;
global_settings {
max_trace_level 5
#if (Use_Radiosity)
radiosity {
brightness 1
recursion_limit 2
always_sample off
normal off
nearest_count 10
}
#end
}
//------------------------------------------------------------------------------
// Camera & lights
//------------------------------------------------------------------------------
background { color rgb 0 }
camera {
location <0, 0, -15>*SCALE
look_at <0, 0, 0>
right <image_width/image_height, 0, 0>
angle 30
}
#declare light_pos = <-1.5, 1.5, 1.5>*SCALE;
light_source {
light_pos
color rgb 3
#if (Soft_Shadows)
area_light <10, 0, 0>, <0, 0, 10>, 5, 5
adaptive 2
jitter
orient circular
#end
fade_distance VDist(<0,0,0>,light_pos)
fade_power 2
}
//------------------------------------------------------------------------------
// SSLT subsurface colors
//------------------------------------------------------------------------------
#local sslt_aqua = <1, 0, 0>;
#local sslt_magenta = <0, 1, 0>;
#local sslt_yellow = <0, 0, 1>;
#local sslt_blue = <1, 1, 0> ;
#local sslt_red = <0, 1, 1>;
#local sslt_green = <1, 0, 1>;
#local sslt_grey = <0, 0, 0>;
//------------------------------------------------------------------------------
// Macro: MakeSphere()
// SSLT Sphere
//------------------------------------------------------------------------------
#macro MakeSphere(clr)
#local AMT = 0.5;
//#local amt = < 1.6732, 1.2806, 0.6947>; // clipka's from subsurface.pov
#local amt = < 0.001, 0.001, 0.001>*AMT;
sphere { 0, 1
texture {
pigment {rgb 0}
finish{
#local AMT = 20;
#local amt = < 0.001, 0.001, 0.001>*AMT;
subsurface { amt, clr }
}
}
texture {
pigment {
bozo
color_map {[0 rgbt 0][1 rgbt 1]}
scale 0.2
turbulence 0.5
rotate RRand(0, 360, R)
}
normal {
bozo
normal_map {
[0 granite 1 scale 10]
[1 agate 0.01 ]
}
turbulence 0.5
scale 0.075
bump_size -5
}
finish {
ambient 0
diffuse 0.6
specular 0.25 roughness 0.02
}
}
interior { ior 1.33 }
}
#end
//------------------------------------------------------------------------------
// The spheres
//------------------------------------------------------------------------------
union {
object { MakeSphere(sslt_green) translate x*2.5 rotate z*360/7*0 }
object { MakeSphere(sslt_aqua) translate x*2.5 rotate z*360/7*1 }
object { MakeSphere(sslt_blue) translate x*2.5 rotate z*360/7*2 }
object { MakeSphere(sslt_magenta) translate x*2.5 rotate z*360/7*3 }
object { MakeSphere(sslt_red) translate x*2.5 rotate z*360/7*4 }
object { MakeSphere(sslt_yellow) translate x*2.5 rotate z*360/7*5 }
object { MakeSphere(sslt_grey) translate x*2.5 rotate z*360/7*6 }
scale SCALE
}
//end file======================================================================
Cheers,
Rob
-------------------------------------------------
www.McGregorFineArt.com
Post a reply to this message
Attachments:
Download 'sslt_spheres.png' (440 KB)
Preview of image 'sslt_spheres.png'
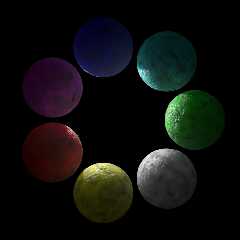
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
I like this, Robert. I have not experimented much with sss, but I am
certainly going to play around with your code for a start.
Thomas
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
"Thomas de Groot" <tDOTdegroot@interDOTnlANOTHERDOTnet> wrote:
> I like this, Robert. I have not experimented much with sss, but I am
> certainly going to play around with your code for a start.
I, too, feel a need to play with this new feature. Nice example!
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
"Bill Pragnell" <bil### [at] hotmail com> wrote:
> "Thomas de Groot" <tDOTdegroot@interDOTnlANOTHERDOTnet> wrote:
> > I like this, Robert. I have not experimented much with sss, but I am
> > certainly going to play around with your code for a start.
>
> I, too, feel a need to play with this new feature. Nice example!
Thanks guys, the documentation's a bit sketchy so I've been trying all sorts of
crazy things with SSLT to see what each parameter actually does. This morning I
discovered that there's a new global_settings param [mm_per_unit], so instead of
using the SCALE for scaling things up 100x to keep the effect subltle:
global_settings {
...
mm_per_unit 1000 // default is 10
}
#declare SCALE = 1;
Not exactly the same but I like this better with a bit more SSLT happening.
-------------------------------------------------
www.McGregorFineArt.com
Post a reply to this message
Attachments:
Download 'sslt_spheres2.png' (431 KB)
Preview of image 'sslt_spheres2.png'
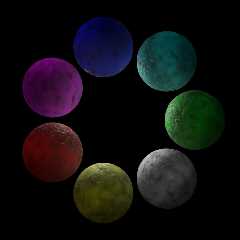
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
I have a funny feeling that I *might* not be seeing this .png image correctly in
my browser window, but I just don't know for sure. ( Firefox v3.6.10, the latest
AFAIK.) What I see is a generally dark image, as well as the bright soft
'crescents' of the spheres, with just a *hint* of color on their dark sides.
Sound correct? (I took the image into my old version of Photoshop, changed *its*
2.2 gamma to 1.0--brightening the spheres' dark sides up nicely--saved it as a
jpeg, and the image looks like what I *think* I should be seeing.) But of
course, the original darker image may be what's intended.
I just want to make sure that I'm seeing proper .png behavior on my end; I
sometimes wonder, given previous (but sporadic) behavior.
Ken
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
> area_light<10, 0, 0>,<0, 0, 10>, 5, 5
> adaptive 2
Do you realise that adaptive 2 tries to use at least a 5x5 aray in your
area_light?
In this case, with or without adaptive, it takes the same amount of time
as there is no adaptive sampling done at all.
nice image by the way. Time to play some more with sslt.
Alain
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
On 09/22/2010 08:44 AM, Robert McGregor wrote:
> Thanks guys, the documentation's a bit sketchy so I've been trying all sorts of
> crazy things with SSLT to see what each parameter actually does. This morning I
> discovered that there's a new global_settings param [mm_per_unit], so instead of
> using the SCALE for scaling things up 100x to keep the effect subltle:
I'm fairly up to date over there reference-wise:
http://wiki.povray.org/content/Documentation:Reference_Section_5.1#Subsurface_Light_Transport
but would like to add something to the tutorial on this feature. I'm
currently working on a new radiosity tutorial, so couldn't possibly add
more at the moment, but if anyone would like to put together a bit of a
demo while you guys are toying around with this new feature, I'd love to
see it and perhaps get it into the docs ... Any takers?
Jim
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
Those balls look very good, I kinda like them. Can you create a version of
1600 pixels width? I would like to have it as desktop background. Thanks.
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
> Those balls look very good, I kinda like them. Can you create a version of
> 1600 pixels width? I would like to have it as desktop background. Thanks.
>
>
You have the code, look at the first post, you can render it yourself at
any resolution you want.
Alain
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
"Robert McGregor" <rob### [at] mcgregorfineart com> wrote:
> More SSLT fun, and maybe I've got this all wrong, but I'm following Jaime's
> wise ignorancia philosophy and this is working for me right now...
>
> Please pardon the code here, but I think it's nice posted all in one spot like
> this:
>
> ...
Appetizing!
:-)
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|
 |