 |
 |
|
 |
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
clipka <ano### [at] anonymous org> wrote:
> (You could achieve the same syntax by having multiplierFunction() return
> an object with an overridden () operator rather than a function, but of
> course that would be cheating.)
That's kind of what the C++ lambda does internally: It puts the captured
variables in some kind of internal struct, which the function then operates
with (similarly to how a class member function operates with the object
using the 'this' pointer). You could achieve a similar behavior by writing
a class, but a lambda achieves this with significantly less effort.
There is a significant difference to a class, though. In this example
std::function<int(int)> can manage *any* lambda function that takes an
int and returns an int, not just ones that have captured a 'multiplier'
variable and perform a "value * multiplier" on it.
If you implemented the functionality of the example I wrote with a
class, you would be fixing the "lambda" function to be only and exactly
a multiplier function that has to be used with exactly your class and
nothing else. std::function<int(int)> has no such limitation. Any function
(be it a static function or a lambda) that takes an int and returns one
will do.
This can make a difference especially with non-templated functions that
take a std::function as parameter. (One advantage of std::function over a
generic functor is that the former does not require for the code that uses
it to be templated and thus doesn't suffer form the few limitations that
templated code has.)
--
- Warp
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
Orchid Win7 v1 <voi### [at] dev null> wrote:
> > You can create anonymous functions. The syntax is:
> >
> > [](int x) { return 2*x; }
> >
> > (Of course that alone won't do anything because you can't call it, as
> > it has no name. However, you can eg. create a variable that represents
> > the function, like: "auto func = [](int x) { return 2*x; };". If you need
> > to eg. return that function from another function, you'll have to use the
> > std::function wrapper. Likewise if you need to give one as parameter to
> > a non-templated or anonymous function.)
> Mmm, interesting.
> So how do you call such a function? Does it just override the usual
> function call notation?
A variable that points to such an anonymous function works like any
function pointer, which means you can call it like a regular function.
Thus if you have, for example:
auto func = [](int x) { return 2*x; };
then you can simply call it like
func(5);
Templates taking a functor as parameter work the same way. You could
have something like:
template<typename T, typename Comparator>
bool compare(const T& value1, const T& value2,
const Comparator& comparatorFunction)
{
return comparatorFunction(value1, value2);
}
In the above example any comparator function will do (a comparator
function is something that behaves like a function taking two parameters
and returns a boolean). This can be a regular function, a functor (a class
that behaves like a function), a lambda, or an object of type std::function.
Thus you can call it eg. like:
compare(1, 3, [](int v1, int v2) { return v1 < v2; })
--
- Warp
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
On 04/22/2012 09:06 AM, Stephen wrote:
> On 22/04/2012 1:38 PM, James Holsenback wrote:
>> On 04/21/2012 06:14 PM, Stephen wrote:
>
>>>
>>> I should have said that I am none the wiser. I still do not know what a
>>> monad is.
>>>
>>
>> Oh that's easy ... it's just the singular form of gonad ... THUD ...
>> couldn't resist ;-)
>
> That calls for a kick in both monads. :-P
>
hmmmm ... nonads (well that's self explanatory) better yet faux-nads ...
someone who thinks that gotta pair. OK I'll stop now ;-)
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
Invisible <voi### [at] dev null> wrote:
> One Haskell VFAQ is "What is a monad?"
http://byorgey.files.wordpress.com/2011/05/monad_tutorial.jpg
--
- Warp
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
>> The question is, does
>
>> multiplierFunction(3)(4)
>
>> or similar yield a 12?
>
> Yes.
Right. So if you implemented all your stuff to take one argument at a
time like this [which would presumably be a little bit of work], IMHO
you could perfectly well call that "curried functions".
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
On 22/04/2012 06:03 PM, Warp wrote:
> That's kind of what the C++ lambda does internally: It puts the captured
> variables in some kind of internal struct, which the function then operates
> with (similarly to how a class member function operates with the object
> using the 'this' pointer). You could achieve a similar behavior by writing
> a class, but a lambda achieves this with significantly less effort.
Oh, so it can capture local variables too? That's even better than I
thought...
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
On 22/04/2012 05:34 PM, clipka wrote:
> Maybe that's what scrambles my mind whenever I read "Haskellists'"
> explanations of stuff: They're so proud that Haskell does it
> automatically for you, that they tend to deny the obvious parallels.
>
> So yes: Currying *is* like writing a function that takes fewer
> arguments, calling the original one and supplying (unchangeable)
> defaults for the missing arguments.
>
> And yes, apparently Haskell provides syntactic sugar for that. Wow. Big
> deal.
>
>> Finally, like I said, currying works backwards too: If a function call
>> happens to return a function as its result, you can just keep appending
>> arguments. "head function_list 7" and all that.
>
> Again, nice-to-have syntactic sugar, but nothing conceptually
> fascinating (as you noted yourself).
You seem to be missing the third point: Function currying allows you to
write code that polymorphically accepts functions with ANY number of
arguments.
That can be a Big Deal.
That said, yeah, of all the things that Haskell does, function currying
is one of the less exciting ones.
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
>> One Haskell VFAQ is "What is a monad?"
>
> http://byorgey.files.wordpress.com/2011/05/monad_tutorial.jpg
Post a reply to this message
Attachments:
Download '960526421_mnnqb-o-1.jpg' (24 KB)
Preview of image '960526421_mnnqb-o-1.jpg'
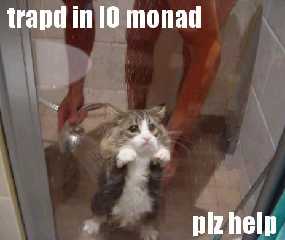
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
On 22/04/2012 6:44 PM, James Holsenback wrote:
> hmmmm ... nonads (well that's self explanatory) better yet faux-nads ...
> someone who thinks that gotta pair. OK I'll stop now ;-)
I think, for everyone's sake, that you had better. ;-)
--
Regards
Stephen
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
On 22/04/2012 08:44 PM, Stephen wrote:
> On 22/04/2012 6:44 PM, James Holsenback wrote:
>> hmmmm ... nonads (well that's self explanatory) better yet faux-nads ...
>> someone who thinks that gotta pair. OK I'll stop now ;-)
>
> I think, for everyone's sake, that you had better. ;-)
Christ knows what happens if I ever try to explain group
homomorphisms... o_O
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |