 |
 |
|
 |
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
"Bald Eagle" <cre### [at] netscape net> wrote:
> "Droj" <803### [at] droj de> wrote:
>
> > > I will try to do some fine tuning as the heart curve still has an inconsistency
> > > where the dip is.
>
> Yeah - not sure if that's the math or the macro.
> It seems to be right at the beginning of the curve, so maybe instead of going
> from 0 - 2pi, maybe start at pi/180 and see if that trims off the cruft.
>...
My suspicion is that this is caused by a problem with the Paramcalc
macro in meshmaker.inc (The SimpleMesh macro in the code below does
not seem to have this problem.)
The code below also shows an alternative way to make the final
functions for the "tube".
// ===== 1 ======= 2 ======= 3 ======= 4 ======= 5 ======= 6 ======= 7
#version 3.7;
global_settings { assumed_gamma 1.0 }
#include "functions.inc" // For f_r()
#include "colors.inc"
#declare TAU = 2*pi;
// ===== 1 ======= 2 ======= 3 ======= 4 ======= 5 ======= 6 ======= 7
#macro SimpleMesh(FnX, FnY, FnZ, MinU, MaxU, MinV, MaxV, SizeI, SizeJ)
#local SpanU = MaxU - MinU;
#local SpanV = MaxV - MinV;
#local LastI = SizeI - 1;
#local LastJ = SizeJ - 1;
#local Vertices = array[SizeI][SizeJ];
#for (I, 0, LastI)
// #local U = MinU + I/LastI*DU;
#local S = I/LastI;
#local U = MinU + S*SpanU;
// #local U = FnU(I/LastI);
#for (J, 0, LastJ)
// #local V = MinV + I/LastJ*DV;
// #local V = FnU(J/LastJ);
#local T = J/LastJ;
#local V = MinV + T*SpanV;
#local Vertices[I][J] =
<FnX(U, V), FnY(U, V), FnZ(U, V)>
;
#end // for
#end // for
mesh {
#for (I, 0, LastI - 1)
#for (J, 0, LastJ - 1)
#local p00 = Vertices[I ][J ];
#local p01 = Vertices[I ][J+1];
#local p10 = Vertices[I+1][J ];
#local p11 = Vertices[I+1][J+1];
triangle { p00, p10, p11 }
triangle { p11, p01, p00 }
#end // for
#end // for
}
#end // macro SimpleMesh
// ===== 1 ======= 2 ======= 3 ======= 4 ======= 5 ======= 6 ======= 7
// Heart curve from https://www.frassek.org
// 0 <= u <= TAU
// Parametric functions for Heart curve and their derivatives:
#declare FnX =
function(u) {
16*pow(sin(u), 3)
}
;
#declare DFnX =
function(u) {
48*pow(sin(u), 2)*cos(u)
}
;
#declare FnY =
function(u) {
13*cos(u) - 5*cos(2*u) - 2*cos(3*u) - cos(4*u)
}
;
#declare DFnY =
function(u) {
-13*sin(u) + 10*sin(2*u) + 6*sin(3*u) + 4*sin(4*u)
}
;
// ===== 1 ======= 2 ======= 3 ======= 4 ======= 5 ======= 6 ======= 7
#declare Nil = 1e-3;
#declare R = 1.2;
// Rotation angle of cross section of tube
#declare AngleFn =
function(u) {
atan2(DFnY(u), DFnX(u)) + pi/2
}
;
union {
SimpleMesh(
function(u, v) { R*cos(v)*cos(AngleFn(u)) + FnX(u) },
function(u, v) { R*cos(v)*sin(AngleFn(u)) + FnY(u) },
function(u, v) { R*sin(v) },
0 + Nil, TAU - Nil,
0 + Nil, TAU - Nil,
200, 16
)
sphere { <FnX(0/2*TAU), FnY(0/2*TAU), 0>, R }
sphere { <FnX(1/2*TAU), FnY(1/2*TAU), 0>, R }
pigment { color Gray50 + Yellow/4 }
translate -20*x
}
// Normalized component functions for the tangent vector
#declare N_DFnX =
function(u) {
DFnX(u)/f_r(DFnX(u), DFnY(u), 0)
}
;
#declare N_DFnY =
function(u) {
DFnY(u)/f_r(DFnX(u), DFnY(u), 0)
}
;
union {
SimpleMesh(
function(u, v) { -R*cos(v)*N_DFnY(u) + FnX(u) },
function(u, v) { +R*cos(v)*N_DFnX(u) + FnY(u) },
function(u, v) { +R*sin(v) },
0 + Nil, TAU - Nil,
0 + Nil, TAU - Nil,
200, 16
)
sphere { <FnX(0/2*TAU), FnY(0/2*TAU), 0>, R }
sphere { <FnX(1/2*TAU), FnY(1/2*TAU), 0>, R }
pigment { color Gray50 + Cyan/4 }
translate +20*x
}
// ===== 1 ======= 2 ======= 3 ======= 4 ======= 5 ======= 6 ======= 7
background { color rgb <0.04, 0.02, 0.06> }
light_source {
-15*x
color Gray50 + Red/2
shadowless
}
light_source {
-15*y
color Gray50 + Green/2
shadowless
}
light_source {
-15*z
color Gray50 + Blue/2
shadowless
}
#declare AR = image_width/image_height;
camera {
orthographic
location -100*z
direction z
right AR*x
up y
sky y
angle 45
}
// ===== 1 ======= 2 ======= 3 ======= 4 ======= 5 ======= 6 ======= 7
--
Tor Olav
http://subcube.com
https://github.com/t-o-k
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
"Tor Olav Kristensen" <tor### [at] TOBEREMOVEDgmail com> wrote:
> "Bald Eagle" <cre### [at] netscape net> wrote:
> > "Droj" <803### [at] droj de> wrote:
> >
> > > > I will try to do some fine tuning as the heart curve still has an
inconsistency
> > > > where the dip is.
> >
> > Yeah - not sure if that's the math or the macro.
> > It seems to be right at the beginning of the curve, so maybe instead of going
> > from 0 - 2pi, maybe start at pi/180 and see if that trims off the cruft.
> >...
>
> My suspicion is that this is caused by a problem with the Paramcalc
> macro in meshmaker.inc (The SimpleMesh macro in the code below does
> not seem to have this problem.)
>
> The code below also shows an alternative way to make the final
> functions for the "tube".
>...
I see now that the SimpleMesh macro is a bit messy.
Here's a cleaned up version:
// ===== 1 ======= 2 ======= 3 ======= 4 ======= 5 ======= 6 ======= 7
#macro SimpleMesh(FnX, FnY, FnZ, MinU, MaxU, MinV, MaxV, SizeI, SizeJ)
#local LastI = SizeI - 1;
#local LastJ = SizeJ - 1;
#local Vertices = array[SizeI][SizeJ];
#local SpanU = MaxU - MinU;
#local SpanV = MaxV - MinV;
#for (I, 0, LastI)
#local U = MinU + I/LastI*SpanU;
#for (J, 0, LastJ)
#local V = MinV + J/LastJ*SpanV;
#local Vertices[I][J] =
<FnX(U, V), FnY(U, V), FnZ(U, V)>
;
#end // for
#end // for
mesh {
#for (I, 0, LastI - 1)
#for (J, 0, LastJ - 1)
#local p00 = Vertices[I ][J ];
#local p01 = Vertices[I ][J+1];
#local p10 = Vertices[I+1][J ];
#local p11 = Vertices[I+1][J+1];
triangle { p00, p10, p11 }
triangle { p11, p01, p00 }
#end // for
#end // for
}
#end // macro SimpleMesh
// ===== 1 ======= 2 ======= 3 ======= 4 ======= 5 ======= 6 ======= 7
--
Tor Olav
http://subcube.com
https://github.com/t-o-k
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
Le 2022-12-24 à 15:33, Droj a écrit :
> "Droj" <803### [at] droj de> wrote:
>> "Bald Eagle" <cre### [at] netscape net> wrote:
>>> "Bald Eagle" <cre### [at] netscape net> wrote:
>>>
>>>> I'm confident that this is a solvable problem.
>>>
>>>
>>> As I said. Success! :)
>>>
>>> Looks like all you have to do is change these two lines:
>>>
>>> I got the atan to give me a "clean" curve, but it was really skinny. "maybe
>>> that's just rotated 90 deg from what it should be...?
>>> So I got rid of the pi/2. No idea _why_ that works... yet.
>>>
>>>
>>> #declare PHI = function (u) {-atan2( FdY(u), FdX(u) ) + pi/2*0};
>>>
>>> #declare F1 = function(u,v) {QY(v)*sin(PHI(u)) + FX(u)};
>>
>> Heureka and congratulations! You solved that 'pain in the backside'.
>>
>> I adapted the POV-script according to the functions above and it looks great!
>> Changing QX to QY in F1 did the trick. And right, the pi/2 produces a
>> very, very flat curve almost like a ribbon.
>>
>> I will try to do some fine tuning as the heart curve still has an inconsistency
>> where the dip is.
>>
>> But all in all it's a beauty and I'm definitely happy.
>>
>> I wish you a Merry Christmas and thank you so much.
>>
>> Oswald
>
> PS:
> Your solution works with simple Lissajous functions, too.
>
> This is a great Christmas present!
>
Personally, for that one, I would have used a sphere_sweep using the
bi_cubic interpolation.
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
"Tor Olav Kristensen" <tor### [at] TOBEREMOVEDgmail com> wrote:
> "Tor Olav Kristensen" <tor### [at] TOBEREMOVEDgmail com> wrote:
> > "Bald Eagle" <cre### [at] netscape net> wrote:
> > > "Droj" <803### [at] droj de> wrote:
> > >
> > > > > I will try to do some fine tuning as the heart curve still has an
inconsistency
> > > > > where the dip is.
> > My suspicion is that this is caused by a problem with the Paramcalc
> > macro in meshmaker.inc (The SimpleMesh macro in the code below does
> > not seem to have this problem.)
> >
> > The code below also shows an alternative way to make the final
> > functions for the "tube".
> >...
>
> I see now that the SimpleMesh macro is a bit messy.
I haven't gone through any of the edited mesh macro code yet, but did some
simple mesh creation code of my own, and the problem was still in the
calculation of that damned angle Phi. It's not very well-behaved, and jumps
around a lot, making adjacent sections of mesh "flip" through the central curve
trace.
I played around with it for quite a while, graphing the values of Phi, and
finally came up with this:
#macro Implicit_CalculatePhi (UU, I_Derivative)
#local Phi = -atan2 (I_Derivative (UU)*UU, abs (UU+E));
// correct for flipping around x=0
#local Phi = select ((Phi+pi/2), Phi+pi, Phi);
#local Phi = select (UU, -Phi, 0, Phi);
Phi
#end
It keeps Phi as a nice, continuous value between +/-pi
So in the loops, instead of calculating Phi, just use:
#local Phi = Implicit_CalculatePhi (UU, I_Derivative);
And that seems to have eliminated all of the problems I've noticed at x0 <= 0
where there are mins and maxes in the curve.
Hopefully this does the trick. I have more curves to plot, and then need to
adapt my macros to parametrics and then the polynomial curves.
Post a reply to this message
Attachments:
Download 'tubecurves.png' (183 KB)
Preview of image 'tubecurves.png'
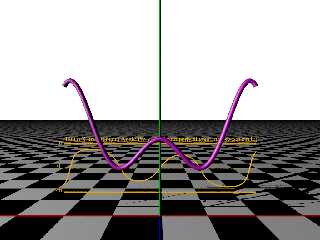
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
> Hopefully this does the trick. I have more curves to plot, and then need to
> adapt my macros to parametrics and then the polynomial curves.
......aaand it's not quite. When the curve is nearly vertical, my select ()
statement hard-codes the Phi to be zero, so maybe I have to do a central
differences type thing and average f(+/-h)
Post a reply to this message
Attachments:
Download 'tubecurves.png' (222 KB)
Preview of image 'tubecurves.png'
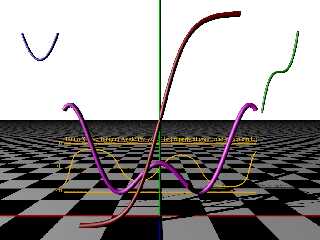
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
"Bald Eagle" <cre### [at] netscape net> wrote:
> "Tor Olav Kristensen" <tor### [at] TOBEREMOVEDgmail com> wrote:
> > "Tor Olav Kristensen" <tor### [at] TOBEREMOVEDgmail com> wrote:
> > > "Bald Eagle" <cre### [at] netscape net> wrote:
> > > > "Droj" <803### [at] droj de> wrote:
> > > >
> > > > > > I will try to do some fine tuning as the heart curve still has an
inconsistency
> > > > > > where the dip is.
>
>
> > > My suspicion is that this is caused by a problem with the Paramcalc
> > > macro in meshmaker.inc (The SimpleMesh macro in the code below does
> > > not seem to have this problem.)
> > >
> > > The code below also shows an alternative way to make the final
> > > functions for the "tube".
> > >...
> >
> > I see now that the SimpleMesh macro is a bit messy.
>
> I haven't gone through any of the edited mesh macro code yet, but did some
> simple mesh creation code of my own, and the problem was still in the
> calculation of that damned angle Phi. It's not very well-behaved, and jumps
> around a lot, making adjacent sections of mesh "flip" through the central curve
> trace.
>
> I played around with it for quite a while, graphing the values of Phi, and
> finally came up with this:
>
> #macro Implicit_CalculatePhi (UU, I_Derivative)
> #local Phi = -atan2 (I_Derivative (UU)*UU, abs (UU+E));
> // correct for flipping around x=0
> #local Phi = select ((Phi+pi/2), Phi+pi, Phi);
> #local Phi = select (UU, -Phi, 0, Phi);
>
> Phi
> #end
>
> It keeps Phi as a nice, continuous value between +/-pi
>
> So in the loops, instead of calculating Phi, just use:
> #local Phi = Implicit_CalculatePhi (UU, I_Derivative);
>
> And that seems to have eliminated all of the problems I've noticed at x0 <= 0
> where there are mins and maxes in the curve.
>
> Hopefully this does the trick. I have more curves to plot, and then need to
> adapt my macros to parametrics and then the polynomial curves.
Bill,
I'm worried that maybe you are making things more complicated than they are.
That's because my tests indicate that atan2(DFnY(u), DFnX(u)) does a good job
calculating the angle of the tangent. One just have to add or subtract pi/2 to
get an angle pi/2 (90 degrees) away from the tangent direction (in the
XY-plane).
I'll try to explain...
If we, in order to simplify things, set the tube radius to 1 and pretend
that POV-Ray has 3D-vector valued functions, then the problem can be
solved like this:
X = function(u) { 16*pow(sin(u), 3) }
Y = function(u) { 13*cos(u) - 5*cos(2*u) - 2*cos(3*u) - cos(4*u) }
pCtr = function3d(u) { <X(u), Y(u), 0> }
d_X = function(u) { 48*pow(sin(u), 2)*cos(u) }
d_Y = function(u) { -13*sin(u) + 10*sin(2*u) + 6*sin(3*u) + 4*sin(4*u) }
vTangent = function3d(u) { <d_X(u), d_Y(u), 0> }
TangentAngle = function(u) { atan2(d_Y(u), d_X(u)) }
OrthAngle = function(u) { TangentAngle(u) + pi/2 } // or TangentAngle(u) - pi/2
We need two vectors that are orthogonal to the tangent of the curve,
that is orthogonal to each other and has unit length. Since this is
a 2D curve in an XY-plane, they can be created like this:
vOrth_1a = function3d(u) { <cos(OrthAngle(u)), sin(OrthAngle(u)), 0> }
vOrth_2 = function3d(u) { <0, 0, +1> } // or <0, 0, -1>
All the points on the surface can now be calculated like this:
pSurface =
function3d(u, v) {
pCtr(u) +
cos(v)*vOrth_1a(u) +
sin(v)*vOrth_2(u)
}
pSurface =
function3d(u, v) {
<X(u), Y(u), 0> +
cos(v)*<cos(OrthAngle(u)), sin(OrthAngle(u)), 0> +
sin(v)*<0, 0, 1>
}
pSurface =
function3d(u, v) {
<X(u), Y(u), 0> +
<cos(v)*cos(OrthAngle(u)), cos(v)*sin(OrthAngle(u)), 0> +
<0, 0, sin(v)>
}
pSurface =
function3d(u, v) {
<
cos(v)*cos(OrthAngle(u)) + X(u),
cos(v)*sin(OrthAngle(u)) + Y(u),
sin(v)
>
}
Here's an alternative way of creating the first orthogonal vector:
vTangentNormalized =
function3d(u) {
<cos(TangentAngle(u)), sin(TangentAngle(u)), 0>
}
vOrth_1b =
function3d(u) {
<-vTangentNormalized(u).y, +vTangentNormalized(u).x, 0>
// or <+vTangentNormalized(u).y, -vTangentNormalized(u).x, 0>
}
vOrth_1b =
function3d(u) {
<-sin(TangentAngle(u)), +cos(TangentAngle(u)), 0>
}
Again the points on the surface can be calculated like this:
pSurface =
function3d(u, v) {
pCtr(u) +
cos(v)*vOrth_1b(u) +
sin(v)*vOrth_2(u)
}
pSurface =
function3d(u, v) {
<X(u), Y(u), 0> +
cos(v)*<-sin(TangentAngle(u)), +cos(TangentAngle(u)), 0> +
sin(v)*<0, 0, 1>
}
pSurface =
function3d(u, v) {
<X(u), Y(u), 0> +
<cos(v)*-sin(TangentAngle(u)), cos(v)*+cos(TangentAngle(u)), 0> +
<0, 0, sin(v)>
}
pSurface =
function3d(u, v) {
<
-cos(v)*sin(TangentAngle(u)) + X(u),
+cos(v)*cos(TangentAngle(u)) + Y(u),
sin(v)
>
}
The normalized tangent can also be calculated like this:
vTangentNormalized =
function3d(u) {
<d_X(u), d_Y(u), 0>/f_r(d_X(u), d_Y(u), 0)
}
Now we have yet another way to create the first orthogonal vector:
vOrth_1c =
function3d(u) {
<-vTangentNormalized(u).y, +vTangentNormalized(u).x, 0>
// or <+vTangentNormalized(u).y, -vTangentNormalized(u).x, 0>
}
vOrth_1c =
function3d(u) {
<-d_Y(u), +d_X(u), 0>/f_r(d_X(u), d_Y(u), 0)
}
The points on the surface can be calculated like this:
pSurface =
function3d(u, v) {
pCtr(u) +
cos(v)*vOrth_1c(u) +
sin(v)*vOrth_2(u)
}
pSurface =
function3d(u, v) {
<X(u), Y(u), 0> +
cos(v)*<-d_Y(u), +d_X(u), 0>/f_r(d_X(u), d_Y(u), 0) +
sin(v)*<0, 0, 1>
}
pSurface =
function3d(u, v) {
<X(u), Y(u), 0> +
cos(v)*<-d_Y(u), +d_X(u), 0>/f_r(d_X(u), d_Y(u), 0) +
<0, 0, sin(v)>
}
pSurface =
function3d(u, v) {
<
-cos(v)*d_Y(u)/f_r(d_X(u), d_Y(u), 0) + X(u),
+cos(v)*d_X(u)/f_r(d_X(u), d_Y(u), 0) + Y(u),
sin(v)
>
}
--
Tor Olav
http://subcube.com
https://github.com/t-o-k
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
Hi TOK, and Merry Christmas :)
I can follow all of your calculations, and indeed, I'm using many of the same
methods.
But I'm curious if you've applied the results of the calculations to generating
the mesh of the function's tubular envelope.
That's where I've encountered the sticking point. Since I'm creating vertices
of the circles sequentially along the curve, the "ordering" of those vertices
matter when I then loop through them to create my triangles. When there's a
sign change or a sudden plummet to zero, I get a severe twist in the tube at
that point.
Maybe you can render the following equations so that I can see that your methods
work, and I indeed (as always) am making things too complicated. You may have
noticed that I DO have that knack. ;)
#declare Function = function (U) {U*U+1}
#declare Derivative = function (U) {2*U}
#declare Function = function (U) {0.4 * cos (U) * (-pow (U, 2) + 3 ) -1}
#declare Derivative = function (U) {0.4 * (pow (U, 2) - 3 )* sin (U) - 0.8 * U *
cos (U)}
#declare Function = function (U) {U*U*U}
#declare Derivative = function (U) {3*U*U}
hyperbolic tangent
#declare Function = function (U) {4 * (sinh (U) / cosh (U)) + 4}
#declare Derivative = function (U) {pow (1/cosh(U), 2)}
"softsign"
#declare Function = function (U) {8*(U/(abs(U)+1))}
#declare Derivative = function (U) {pow (1/(abs(x)+1), 2)}
I came up with a bit of a hacky solution that seems to work for ALL of these. I
keep track of the Phi value for each circle, and if I'm at x=0, I just use the
value from the previous circle, and I get nice, continuous results.
After calculating Phi, I just invoke:
#local Phi = (abs (UU)<E ? LastPhi : Phi);
Here's hoping you prove me wrong with a super simple solution. :)
- BW
Post a reply to this message
Attachments:
Download 'tubecurves.png' (217 KB)
Preview of image 'tubecurves.png'
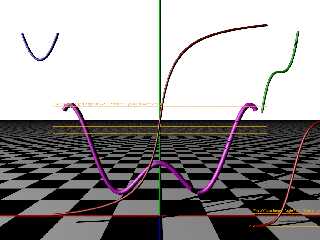
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
"Bald Eagle" <cre### [at] netscape net> wrote:
> Hi TOK, and Merry Christmas :)
Thank you Bill =)
Merry Christmas to you too !
> I can follow all of your calculations, and indeed, I'm using many of the same
> methods.
Ok. That's good.
> But I'm curious if you've applied the results of the calculations to generating
> the mesh of the function's tubular envelope.
Yes, I did that. And it looks ok when I'm using my own mesh macro.
But when I use meshmaker.inc it does not look so good.
> That's where I've encountered the sticking point. Since I'm creating vertices
> of the circles sequentially along the curve, the "ordering" of those vertices
> matter when I then loop through them to create my triangles. When there's a
> sign change or a sudden plummet to zero, I get a severe twist in the tube at
> that point.
>
> Maybe you can render the following equations so that I can see that your methods
> work, and I indeed (as always) am making things too complicated. You may have
> noticed that I DO have that knack. ;)
>...
Ok, but please note that Wolfram Alpha does not agree with you on the
derivatives of the last two. Here's how I wrote them:
#declare FnX = function(u) { u };
#declare DFnX = function(u) { 1 };
#declare Fn_Y = array[5];
#declare DFn_Y = array[5];
#declare I = 0;
#declare Fn_Y[I] = function(u) { pow(u, 2) + 1 };
#declare DFn_Y[I] = function(u) { 2*u };
#declare I = I + 1;
#declare Fn_Y[I] = function(u) { 0.4*cos(u)*(-pow(u, 2) + 3) - 1 };
#declare DFn_Y[I] = function(u) { 0.4*(pow(u, 2) - 3)*sin(u) - 0.8*u*cos(u) };
#declare I = I + 1;
#declare Fn_Y[I] = function(u) { u*u*u };
#declare DFn_Y[I] = function(u) { 3*u*u };
#declare I = I + 1;
#declare Fn_Y[I] = function(u) { 4*sinh(u)/cosh(u) + 4 };
#declare DFn_Y[I] = function(u) { 16*pow(cosh(u), 2)/pow(cosh(2*u) + 1, 2) };
#declare I = I + 1;
#declare Fn_Y[I] = function(u) { 8*u/(abs(u) + 1) };
#declare DFn_Y[I] = function(u) { 8/pow(abs(u) + 1, 2) };
I ran all these through the SimpleMesh macro. See the attached image for
the results. I can post the complete source for that if you're interested.
> I came up with a bit of a hacky solution that seems to work for ALL of these. I
> keep track of the Phi value for each circle, and if I'm at x=0, I just use the
> value from the previous circle, and I get nice, continuous results.
>
> After calculating Phi, I just invoke:
> #local Phi = (abs (UU)<E ? LastPhi : Phi);
I can not understand why that should be necessary. - But it could be that
it's me that's missing something...
> Here's hoping you prove me wrong with a super simple solution. :)
It's not super simple, but perhaps less complicated ;)
--
Tor Olav
http://subcube.com
https://github.com/t-o-k
Post a reply to this message
Attachments:
Download 'tube_curves.png' (121 KB)
Preview of image 'tube_curves.png'
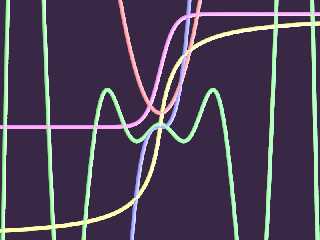
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
"Tor Olav Kristensen" <tor### [at] TOBEREMOVEDgmail com> wrote:
> I ran all these through the SimpleMesh macro. See the attached image for
> the results. I can post the complete source for that if you're interested.
Yes, that would be interesting to look over.
I'll post my own code here, and maybe you can spot why it's not working with
just a simple Phi calculation.
> I can not understand why that should be necessary. - But it could be that
> it's me that's missing something...
I can only imagine I have some minor error somewhere that gets magnified
later....
All I'm doing is storing the vertices of the tilted circle in a 2D array, and
then moving along the curve and storing those circles. Then I travel around the
stored points in the circle array and make quads with 2 triangles. Standard
stuff. Looks great in the positive quadrant/octant and in the smooth parts of
the negative. So it's odd that it's not working well at specific points, and my
graph of the Phi values was so discontinuous.
Bear in mind that I've only done this for the implicit functions, not 3d the
parametrics. But I should get this sorted out and see what the problem is
before I go any further.
Thanks as always for your clear mind, sharp eyes, and _lightning fast_ code
generation!
- BW
Post a reply to this message
Attachments:
Download 'tubecurves.pov.txt' (12 KB)
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
"Bald Eagle" <cre### [at] netscape net> wrote:
> "Tor Olav Kristensen" <tor### [at] TOBEREMOVEDgmail com> wrote:
>...
> I'll post my own code here, and maybe you can spot why it's not working with
> just a simple Phi calculation.
I have had a thorough look at your code and modified it. You can find it here:
Newsgroup: povray.text.scene-files
Subject: Rewrite of tubecurves.pov (2D function to 3D tube)
Date: 2022-12-27 22:37:15
From: Tor Olav Kristensen
http://news.povray.org/povray.text.scene-files/thread/%3Cweb.63abb9396dfe9e73d6142c8889db30a9%40news.povray.org%3E
> > I can not understand why that should be necessary. - But it could be that
> > it's me that's missing something...
>
> I can only imagine I have some minor error somewhere that gets magnified
> later....
Sorry, but I have not found out exactly where your problem is.
> All I'm doing is storing the vertices of the tilted circle in a 2D array, and
> then moving along the curve and storing those circles. Then I travel around the
> stored points in the circle array and make quads with 2 triangles. Standard
> stuff.
> ...
Yes, that's a sensible way to do it.
>...
> Looks great in the positive quadrant/octant and in the smooth parts of
> the negative. So it's odd that it's not working well at specific points, and my
> graph of the Phi values was so discontinuous.
>
> Bear in mind that I've only done this for the implicit functions, not 3d the
> parametrics. But I should get this sorted out and see what the problem is
> before I go any further.
>
> Thanks as always for your clear mind, sharp eyes, and _lightning fast_ code
> generation!
Hehe, no problem.
I had previously done some work related to this topic; with parametric
functions in 3D, so I already had some code to start with.
If you're interested, you can have a look at the "Trefoil Knot Tube"
example here:
https://github.com/t-o-k/scikit-vectors_examples
https://github.com/t-o-k/scikit-vectors_examples/blob/master/Trefoil_Knot_Tube.pdf
Sometimes GitHub fails to render PDF-files and Jupyter Notbook files (.ipynb).
If that happens, just wait a litte and then try again.
--
Tor Olav
http://subcube.com
https://github.com/t-o-k
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|
 |