|
 |
I am having issues in the following code. The cylinder segment boxes
appear to be clipped and hollow, but I'm not actually using `clipped_by`
anywhere. Does anyone have a clue what is happening? Thanks.
Mike
//--------------------------------------------------------------Table
#declare Muns_cen_table = array[11][6]
{
// h V C R G B
{ 0, 0, 0, 0/255, 0/255, 0/255},
{ 0, 1, 0, 28/255, 28/255, 28/255},
{ 0, 2, 0, 48/255, 48/255, 48/255},
{ 0, 3, 0, 70/255, 70/255, 70/255},
{ 0, 4, 0, 97/255, 97/255, 97/255},
{ 0, 5, 0, 124/255, 124/255, 124/255},
{ 0, 6, 0, 150/255, 150/255, 150/255},
{ 0, 7, 0, 179/255, 179/255, 179/255},
{ 0, 8, 0, 203/255, 203/255, 203/255},
{ 0, 9, 0, 232/255, 232/255, 232/255},
{ 0, 10, 0, 255/255, 255/255, 255/255}
}
//--------------------------------------------------------------Parameters
#macro SetClock(StartTime, EndTime)
#local R = (clock - StartTime)/(EndTime - StartTime);
#local S = min(max(0, R), 1);
S
#end
#declare Muns_slice_angle = 360/40;
#declare Muns_gap_width = 1/40; // this is actually 1/2 the true gap
#declare Muns_cam_view = 3; // 0 = isometric; 1 = overhead; 2 = front
or side; 3 = custom
#declare Muns_hue_adjust = Muns_slice_angle * 4;
#declare Muns_time_spin = SetClock(0/3, 1/3);
#declare Muns_time_wipe = SetClock(1/3, 2/3);
#declare Muns_time_tilt = SetClock(2/3, 3/3);
#declare Muns_color_clamp = 0.9;
//--------------------------------------------------------------CSG objects
#local Muns_coo_count = 0;
#local Muns_coo_max = 11;
#while (Muns_coo_count < Muns_coo_max)
#local Muns_param_h = Muns_cen_table[Muns_coo_count][0] * 360/100;
#local Muns_param_V = Muns_cen_table[Muns_coo_count][1] - 5;
#local Muns_param_C = Muns_cen_table[Muns_coo_count][2]/2;
#local Muns_param_r = Muns_cen_table[Muns_coo_count][3];
#local Muns_param_g = Muns_cen_table[Muns_coo_count][4];
#local Muns_param_b = Muns_cen_table[Muns_coo_count][5];
#local Muns_coo_RGB = <Muns_param_r,Muns_param_g,Muns_param_b> *
Muns_color_clamp;
difference
{
cylinder
{
-y * (1/2 - Muns_gap_width),
+y * (1/2 - Muns_gap_width),
1/2 - Muns_gap_width
translate +y * Muns_param_V
rotate +y * Muns_param_h
}
intersection
{
plane {+x, 0}
plane {-x, 0 rotate +y * Muns_time_wipe * 180}
rotate -y * Muns_hue_adjust
}
pigment {color srgb Muns_coo_RGB}
finish {emission 0.3 diffuse 1}
}
#local Muns_coo_count = Muns_coo_count + 1;
#end
Post a reply to this message
Attachments:
Download 'munsell_srgb_example.png' (179 KB)
Preview of image 'munsell_srgb_example.png'
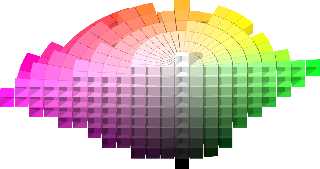
|
 |
|
 |
Le 19-02-19 à 00:23, Mike Horvath a écrit :
> On 2/18/2019 7:55 AM, Mike Horvath wrote:
>> Better if I should attach the whole scene, no?
>>
>>
>> Mike
>
> Performance is really poor in this scene. Each 600x600px frame of my
> test animation takes about 5 minutes to render on my machine. I am doing
> 120 frames. I guess I need to add some `bounded_by` statements in there
> somewhere...
>
>
> Mike
In this case, you have a difference of cylinders intersected by some
planes. Each block may end up with a bounding box large enough to
contain the whole outer cylinder. This is a case where manually bounding
with a tight box or a sphere can help.
Do a test with only a small subset of the blocks, using only the
difference and intersection, where each part is paired with a box made
from the max_extent() and min_extent() for that block.
If there is much unused space, then, you may try using some manual bounding.
This may be your critical part :
intersection{
plane {+x, -Muns_gap_width rotate +y * Muns_slice_angle/2}
plane {-x, -Muns_gap_width rotate -y * Muns_slice_angle/2}
difference{
cylinder{-y*(1/2 -Muns_gap_width),+y*(1/2-Muns_gap_width),Muns_param_C
+ (1/2 - Muns_gap_width)}
cylinder{-y*(1/2),+y*(1/2),Muns_param_C -(1/2 -Muns_gap_width)}
}
translate +y * Muns_param_V
rotate +y * Muns_param_h
}
Post a reply to this message
|
 |