|
 |
This is a planet generator macro which I wrote for fun, using patterned
textures. I don't know if the picture will actually make it through, since
I use the newsreader Pan, which doesn't have any native binary posting
capabilities (anyone know any good binary-posting newsreader for Linux ?),
but I'll attach the macro before it - it's only a few seconds render with
atmoshpere disabled.
No doubt there's plenty to improve here, but this was my first serious
try with making my own texture (or a macro, for that matter)...
The macro is as follows:
*** BEGIN PLANET.INC ***
#include "rand.inc"
#include "functions.inc"
#macro planet(rseed, renderair, renderclouds, renderlights, dayvector)
#local r = seed(rseed);
#local xoff = SRand(r) * 100;
#local yoff = SRand(r) * 100;
#local zoff = SRand(r) * 100;
#local sunx = dayvector.x;
#local suny = dayvector.y;
#local sunz = dayvector.z;
// Sea types
#local seanormal = normal {
bozo
slope_map {
[0 <0,0>]
[0.5 <0.01, 0.5>]
[0.5 <0.01,-0.5>]
[1 <0,0>]
}
warp {
turbulence 2
}
scale 0.001
}
#local sea = texture {
pigment { color rgb <0,0,1> }
normal { seanormal }
}
#local coast = texture {
pigment { color rgb <0,0.5,0.5> }
normal { seanormal }
}
// Landscape types
#local landnormal = normal {
bozo
slope_map {
[0 <0,0> ]
[0.3 <0.3,0.3>]
[0.4 <0.5,0.5>]
[0.5 <0.6,0>]
[0.6 <0.5,-0.5>]
[0.7 <0.3,-0.3>]
[1 <0,0>]
}
warp {
turbulence 1
}
scale 0.01
}
// How many iterations should the continent generator go through ?
#local iterations = 10;
// Our fine continent generator. Works surprisingly well.
#local surfacefunction = function(x,y,z,xoffset,yoffset,zoffset) {
0
#local i = 0;
#local p = 1;
#while (i < iterations)
+ (f_bozo(((x * pow(10,i)) + xoffset), ((y * pow(10,i)) + yoffset),
((z * pow(10,i)) + zoffset)) * (pow(0.1, i) * 0.9))
#local i = i + 1;
#if (i < iterations)
#else
+ (f_bozo(((x * pow(10,i)) + xoffset), ((y * pow(10,i)) + yoffset),
((z * pow(10,i)) + zoffset)) * (pow(0.1, i) * 0.1))
#end
#end
}
// This function decides if a given point should have a city by it's location.
// Returns 0 if not, 1 if yes.
#local cityspot = function(x,y,z) {
select(((surfacefunction(x,y,z, xoff, yoff, zoff) * 0.5 + f_bozo(x * 10000 - yoff,
y * 10000 - zoff, z * 10000 - xoff) * 0.4 + f_bozo((x + xoff) *
1000000, (y + yoff) * 1000000, (z + zoff) * 1000000) * 0.1) -
0.5),1,0,0)
}
// Normalize the vector
#local vlen = function(x1,y1,z1) {
sqrt(x1*x1 + y1*y1 + z1*z1)
}
#local vnormalx = function(x1,y1,z1) {
x1 / vlen(x1,y1,z1)
}
#local vnormaly = function(x1,y1,z1) {
y1 / vlen(x1,y1,z1)
}
#local vnormalz = function(x1,y1,z1) {
z1 / vlen(x1,y1,z1)
}
// Dot the vector
#local vdotter = function(x1,y1,z1,x2,y2,z2) {
x1*x2 + y1*y2 + z1*z2
}
// Calculate the angle
#local vangle = function(x1,y1,z1,x2,y2,z2) {
degrees(acos(min(1,vdotter(vnormalx(x1,y1,z1),vnormaly(x1,y1,z1),
vnormalz(x1,y1,z1),vnormalx(x2,y2,z2), vnormaly(x2,y2,z2),
vnormalz(x2,y2,z2)))))
}
}
}
// The function which calculates if a given point is at the night side
of the planet. // Returns 0 if we're not, 1 if we are. #local nightside
= function(x1,y1,z1,x2,y2,z2) {
select(((90 - vangle(x1,y1,z1,x2,y2,z2)) - surfacefunction(x1*5000,
y1*5000, z1*5000, xoff * -3234, yoff * -3234, zoff * -3234) * 5)
,1,0,0)
}
// The function which calculates if a given point should be a city (with lights) or
not.
// Returns 0 if not, 1 if yes.
#local city = function(x1,y1,z1,x2,y2,z2) {
min(nightside(x1,y1,z1,x2,y2,z2),cityspot(x1,y1,z1))
}
#local forest1 = texture {
pigment { color rgb <0,0.8,0> }
normal { landnormal }
}
#if (renderlights > 0)
#local citytex = texture {
pigment { color rgb <1,0.8,0.05> }
finish { ambient 0.6 }
}
#local forest = texture {
function {
city(x,y,z,sunx,suny,sunz)
}
texture_map {
[0.0 forest1]
[0.5 forest1]
[0.5 citytex]
[1.0 citytex]
}
}
#else
#local forest = texture { forest1 }
#end
#local desert = texture {
pigment { color rgb <255/255,236/255,95/255>}
normal { landnormal }
}
#local polar_icecaps = texture {
pigment { color rgb <0.9,0.9,0.9> }
finish {
brilliance 0.4
}
}
// Continent texture, composed of landscapes
#local land = texture {
function {1 - (abs(y) / 3) }
texture_map {
[0.0 polar_icecaps]
[0.3 polar_icecaps]
[0.33 forest]
[0.8 forest]
[0.85 desert]
[0.90 desert]
[0.95 forest]
[1.0 forest]
}
warp {
turbulence 0.5
}
}
// Atmosphere types
#local airnormal = normal {
bozo
noise_generator 2
slope_map {
[0.0 <0,0>]
[0.3 <0,0.5>]
[0.9 <0.5,0.5>]
[1.0 <0.1,0>]
}
warp {
turbulence 2
}
}
#local clouds = texture {
pigment {
color rgb <1,1,1>
}
normal { airnormal }
finish {
brilliance 0.5
}
}
#local clear = texture {
pigment {
color rgbt <1,1,1,1>
}
// normal { airnormal }
finish {
brilliance 0.5
}
}
// Cloud layer
#local cloudlayer = texture {
bozo
noise_generator 2
warp {
turbulence 2
}
translate <SRand(r) * 100, SRand(r) * 100, SRand(r) * 100>
texture_map {
[0.0 clear]
[0.3 clear]
[0.9 clouds]
[1.0 clouds]
}
}
// Atmosphere density function
#local fd = function(x,y,z) {
max(0, min(1, pow( (1 - (10 * (sqrt(x*x + y*y + z*z) - 3)) ),2 ) ))
// max(0, min(1, ( 1 - (1 * pow((sqrt(x*x + y*y + z*z) - 3) ,2 )) )) )
}
// Atmpshere material
#local atmo = material {
texture { pigment { color rgbt <0,0,0,1> } }
interior {
media {
density {
function {
fd(x,y,z)
}
}
scattering {
4, <0.1,0.1,0.5>
}
}
}
}
/*
// How many iterations should the continent generator go through ?
#local iterations = 10;
// Our fine continent generator. Works surprisingly well.
#local surfacefunction = function(x,y,z,xoffset,yoffset,zoffset) {
0
#local i = 0;
#local p = 1;
#while (i < iterations)
+ (f_bozo(((x * pow(10,i)) + xoffset), ((y * pow(10,i)) + yoffset), ((z *
pow(10,i)) + zoffset))
* (pow(0.1, i) * 0.9))
#local i = i + 1;
#if (i < iterations)
#else
+ (f_bozo(((x * pow(10,i)) + xoffset), ((y * pow(10,i)) + yoffset), ((z *
pow(10,i)) + zoffset))
* (pow(0.1, i) * 0.1))
#end
#end
} */
// The planet surface
#local surface = texture {
/*
#local xoffset = SRand(r) * 100;
#local yoffset = SRand(r) * 100;
#local zoffset = SRand(r) * 100; */
function {
surfacefunction(x,y,z,xoff,yoff,zoff)
}
texture_map {
[0.0 sea]
[0.5 sea]
[0.6 coast]
[0.6 land ]
[1.0 land]
}
}
// The planet and it's atmosphere
union {
// The planet
sphere { <0,0,0>, 3 texture { surface } }
#if (renderclouds > 0)
// Cloud layer
sphere { <0,0,0>, 3.01 texture { cloudlayer } hollow }
#end
#if (renderair > 0)
// Atmosphere
sphere { <0,0,0>, 3.1 material { atmo } hollow }
#end
// Dummy object so we get no warnings if we only render the planet itself
sphere { <0,0,0>,1 pigment { color rgb <1,1,1> } }
}
#end
*** END PLANET.INC ***
Post a reply to this message
Attachments:
Download 'planet.png' (126 KB)
Preview of image 'planet.png'
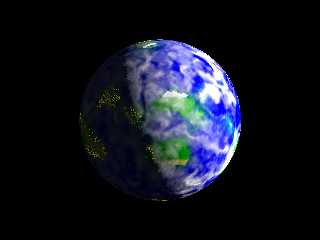
|
 |