 |
 |
|
 |
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
In a scene I am working on, I am building it up with just plain primitive
boxes but when I have the layout all done, I'd like to make them isosurfaces
so I can deform them with noise or pigment functions. I couldn't figure out
an easier way than make a macro that takes the same vectors that define the
box, plus some other parameters, resulting in an isosurface.
This may not be usefull for anyone else but me (meaning, it works on the
scale I'm working with, your results may vary... and it's not tested
heavily), but here's the macro and sample output.
// requires the standard f_rounded_box function
#include "functions.inc"
#macro isoBox (vB1, vB2, fRad, vT1, fDeform, fDeformMagnitude, fAccuracy,
fMaxGradient)
// Paramters:
// vB1 = box vector 1
// vB2 = box vector 2
// fRad = radius for f_rounded_box
// vT1 = deformation translate vector (moves the function away from the
origin)
// fDeform = function to deform the box by
// fDeformMagnitude = scale factor for the deformation function
// fAccuracy = isosurface accuracy
// fMaxGradient = isosurface max_gradient
// X, Y, Z are scales for f_rounded_box
#local X = abs(vB1.x - vB2.x);
#local Y = abs(vB1.y - vB2.y);
#local Z = abs(vB1.z - vB2.z);
// translate the box so it's back where the
// box vectors would place it (f_rounded_box
// places it at the origin it seems)
#local tX = (vB1.x + vB2.x) / 2;
#local tY = (vB1.y + vB2.y) / 2;
#local tZ = (vB1.z + vB2.z) / 2;
#local vTranslate = <tX, tY, tZ>;
// amount to translate the deform function by
#local dX = vT1.x;
#local dY = vT1.y;
#local dZ = vT1.z;
// possibly make this a vector passed as a parameter
// so that each componant can be applied separatelt.
// not sure what to call it, but I use it to smooth out
// the resulting isosurface.
#local dSmooth = 0.33;
isosurface {
function {
f_rounded_box(x, y, z, fRad, X/2, Y/2, Z/2)-
fDeform( (x+dX)*dSmooth, (y+dY)*dSmooth,
(z+dZ)*dSmooth).x*fDeformMagnitude
}
// this contained_by seems adequate for my needs.
contained_by { box { -(max(X, Y, Z)-fRad*2), max(X, Y, Z)+fRad*2 } }
accuracy fAccuracy
max_gradient fMaxGradient
translate vTranslate
}
#end
// example usage:
#declare fnPig2 = function {
pigment {
cells
color_map {
[0.0 rgb 0.0]
[0.3 rgb 1.0]
[0.7 rgb 0.8]
[0.9 rgb 0.3]
}
scale <1, 1, 1> * 0.0085
}
}
object {
isoBox(
<0.085, .085*2, 0.085>,
<-0.085, 0, -0.085>, 0.005
<0, 0, 0>,
fnPig2, 0.01285, 0.001, 4)
pigment {rgb 0.8}
}
Post a reply to this message
Attachments:
Download 'isobox3.png' (29 KB)
Preview of image 'isobox3.png'
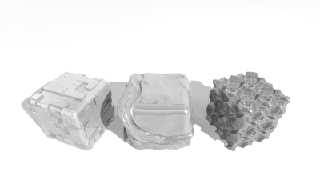
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
On Fri, 17 Nov 2006 15:13:15 -0500, Ross wrote:
> In a scene I am working on, I am building it up with just plain primitive
> boxes but when I have the layout all done, I'd like to make them isosurfaces
> so I can deform them with noise or pigment functions. I couldn't figure out
> an easier way than make a macro that takes the same vectors that define the
> box, plus some other parameters, resulting in an isosurface.
>
> This may not be usefull for anyone else but me (meaning, it works on the
> scale I'm working with, your results may vary... and it's not tested
> heavily), but here's the macro and sample output.
>
>
> // requires the standard f_rounded_box function
> #include "functions.inc"
>
> #macro isoBox (vB1, vB2, fRad, vT1, fDeform, fDeformMagnitude, fAccuracy,
> fMaxGradient)
> // Paramters:
> // vB1 = box vector 1
> // vB2 = box vector 2
> // fRad = radius for f_rounded_box
> // vT1 = deformation translate vector (moves the function away from the
> origin)
> // fDeform = function to deform the box by
> // fDeformMagnitude = scale factor for the deformation function
> // fAccuracy = isosurface accuracy
> // fMaxGradient = isosurface max_gradient
>
> // X, Y, Z are scales for f_rounded_box
> #local X = abs(vB1.x - vB2.x);
> #local Y = abs(vB1.y - vB2.y);
> #local Z = abs(vB1.z - vB2.z);
>
> // translate the box so it's back where the
> // box vectors would place it (f_rounded_box
> // places it at the origin it seems)
> #local tX = (vB1.x + vB2.x) / 2;
> #local tY = (vB1.y + vB2.y) / 2;
> #local tZ = (vB1.z + vB2.z) / 2;
> #local vTranslate = <tX, tY, tZ>;
>
> // amount to translate the deform function by
> #local dX = vT1.x;
> #local dY = vT1.y;
> #local dZ = vT1.z;
>
> // possibly make this a vector passed as a parameter
> // so that each componant can be applied separatelt.
> // not sure what to call it, but I use it to smooth out
> // the resulting isosurface.
> #local dSmooth = 0.33;
>
> isosurface {
> function {
> f_rounded_box(x, y, z, fRad, X/2, Y/2, Z/2)-
> fDeform( (x+dX)*dSmooth, (y+dY)*dSmooth,
> (z+dZ)*dSmooth).x*fDeformMagnitude
> }
>
> // this contained_by seems adequate for my needs.
> contained_by { box { -(max(X, Y, Z)-fRad*2), max(X, Y, Z)+fRad*2 } }
> accuracy fAccuracy
> max_gradient fMaxGradient
>
> translate vTranslate
> }
> #end
>
> // example usage:
> #declare fnPig2 = function {
> pigment {
> cells
> color_map {
> [0.0 rgb 0.0]
> [0.3 rgb 1.0]
> [0.7 rgb 0.8]
> [0.9 rgb 0.3]
> }
> scale <1, 1, 1> * 0.0085
> }
> }
>
> object {
> isoBox(
> <0.085, .085*2, 0.085>,
> <-0.085, 0, -0.085>, 0.005
> <0, 0, 0>,
> fnPig2, 0.01285, 0.001, 4)
> pigment {rgb 0.8}
> }
0:00:00 Parsing
File: isobox_macro_posting.pov Line: 30
File Context (5 lines):
// vB1 = box vector 1
// vB2 = box vector 2
// fRad = radius for f_rounded_box
// vT1 = deformation translate vector (moves the function away from the
origin
Parse Error: Expected 'object', undeclared identifier 'origin' found instead
Total Scene Processing Times
Parse Time: 0 hours 0 minutes 0 seconds (0 seconds)
Photon Time: 0 hours 0 minutes 0 seconds (0 seconds)
Render Time: 0 hours 0 minutes 0 seconds (0 seconds)
Total Time: 0 hours 0 minutes 0 seconds (0 seconds)
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
news: pan.2006.11.18.17.26.29.215251@localhost...
> Parse Error: Expected 'object', undeclared identifier 'origin' found
instead
it is a broken in line comment (//)
the post broke the line (in the parameters at the beginning of the code)
> // vT1 = deformation translate vector (moves the function away from the
> origin)
(guess if it will be broken again)
just comment "origin" as well or delete the carriage return and the >
character
Marc
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
Very nice little macro, Ross! Thank you!!
Thomas
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
"Marc" <jac### [at] wanadoo fr> wrote in message
news:455fa160@news.povray.org...
>
> news: pan.2006.11.18.17.26.29.215251@localhost...
> > Parse Error: Expected 'object', undeclared identifier 'origin' found
> instead
>
> it is a broken in line comment (//)
> the post broke the line (in the parameters at the beginning of the code)
> > // vT1 = deformation translate vector (moves the function away from the
> > origin)
> (guess if it will be broken again)
>
> just comment "origin" as well or delete the carriage return and the >
> character
>
> Marc
>
>
Yep, thanks for clearing that up Marc
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|
 |