|
 |
Hi,
I was looking through old files for something else and came across this scene.
Since I can't remember if I ever posted it or not, I am doing so now!
The basic idea is that you have a cuboid. The longest dimension gets split
somewhere along its length. The algorithm then evaluates the resulting cuboids
and performs the same operation over and over again. In this scene the boxes are
inset by a certain thickness at each iteration, and they are drawn with polar
geometry using cylinders, tori and spheres.
Sorry about the lack of useful comments in the code ':|
(If your system is capable of handling shaders, here's a real time 2D
implementation: https://www.shadertoy.com/view/XlV3DW)
~Sam
Source for this file:
/*
BoxDivideB.pov
2016-2021 Samuel B.
+fn +f +a0.3 +am2 +r1
*/
#version 3.7;
#include "math.inc"
#include "shapes.inc"
#macro Lerp(A, B, C) A*(1-C) + B*C #end
// I believe Rune made this
#macro Z_Align( Normal_vector, Z_vector )
#local ObjectZ = vnormalize( Z_vector );
#local ObjectY = VPerp_Adjust( Normal_vector, Z_vector );
#local ObjectX = vcross( ObjectY, ObjectZ );
transform{ Shear_Trans( ObjectX, ObjectY, ObjectZ ) }
#end
global_settings{
assumed_gamma 1.0
#if(1)
radiosity{
count 1
error_bound .1
recursion_limit 1
nearest_count 10
normal on
}
#end
}
#default{ finish{ambient 0} }
#declare Seed = seed(1002);
#declare SunDir = <-1, .5, -.25>;
#declare RGBSun = srgb .74*<2, 1.95, 1.8>;
#declare RGBSky = rgb <.1, .2, .4>;
#declare CamPos = <0, 4, -8>;
camera{
right x*16/9 up y
location CamPos
look_at .67*y
angle 60/2.7
aperture .25 focal_point y/2 blur_samples 20 variance 0
}
// sunlight
#if(1)
light_source{
SunDir*1e7, RGBSun
#if(1)
#declare ALRes = 2;
#declare ALSize = .03*1e7;
area_light x*ALSize, z*ALSize, ALRes, ALRes
jitter
adaptive 1
area_illumination
#end
}
#end
#if(1)
sky_sphere{
pigment{
spherical scale 2 translate z
poly_wave 2
Z_Align(-y, SunDir)
color_map{
[0 RGBSky]
[1 RGBSun]
}
}
}
#end
#macro WireBox(C1, C2, Thk)
cylinder{<C1.x, C1.y, C1.z>, <C2.x, C1.y, C1.z>, Thk}
cylinder{<C1.x, C1.y, C2.z>, <C2.x, C1.y, C2.z>, Thk}
cylinder{<C1.x, C2.y, C1.z>, <C2.x, C2.y, C1.z>, Thk}
cylinder{<C1.x, C2.y, C2.z>, <C2.x, C2.y, C2.z>, Thk}
cylinder{<C1.x, C1.y, C1.z>, <C1.x, C2.y, C1.z>, Thk}
cylinder{<C2.x, C1.y, C1.z>, <C2.x, C2.y, C1.z>, Thk}
cylinder{<C1.x, C1.y, C2.z>, <C1.x, C2.y, C2.z>, Thk}
cylinder{<C2.x, C1.y, C2.z>, <C2.x, C2.y, C2.z>, Thk}
cylinder{<C1.x, C1.y, C1.z>, <C1.x, C1.y, C2.z>, Thk}
cylinder{<C1.x, C2.y, C1.z>, <C1.x, C2.y, C2.z>, Thk}
cylinder{<C2.x, C1.y, C1.z>, <C2.x, C1.y, C2.z>, Thk}
cylinder{<C2.x, C2.y, C1.z>, <C2.x, C2.y, C2.z>, Thk}
sphere{<C1.x, C1.y, C1.z>, Thk}
sphere{<C2.x, C1.y, C1.z>, Thk}
sphere{<C1.x, C2.y, C1.z>, Thk}
sphere{<C2.x, C2.y, C1.z>, Thk}
sphere{<C1.x, C1.y, C2.z>, Thk}
sphere{<C2.x, C1.y, C2.z>, Thk}
sphere{<C1.x, C2.y, C2.z>, Thk}
sphere{<C2.x, C2.y, C2.z>, Thk}
#end
#macro WireBox_Wedge(C1, C2, Thk)
#local A1 = degrees(C1.x);
#local A2 = degrees(C2.x);
#local Wedge_ = object{Wedge(A2-A1) rotate y*A1}
torus{C1.z, Thk clipped_by{Wedge_} translate y*C1.y}
torus{C1.z, Thk clipped_by{Wedge_} translate y*C2.y}
torus{C2.z, Thk clipped_by{Wedge_} translate y*C1.y}
torus{C2.z, Thk clipped_by{Wedge_} translate y*C2.y}
cylinder{<0, C1.y, C1.z>, <0, C2.y, C1.z>, Thk rotate y*A1}
cylinder{<0, C1.y, C1.z>, <0, C2.y, C1.z>, Thk rotate y*A2}
cylinder{<0, C1.y, C2.z>, <0, C2.y, C2.z>, Thk rotate y*A1}
cylinder{<0, C1.y, C2.z>, <0, C2.y, C2.z>, Thk rotate y*A2}
cylinder{<0, C1.y, C1.z>, <0, C1.y, C2.z>, Thk rotate y*A1}
cylinder{<0, C1.y, C1.z>, <0, C1.y, C2.z>, Thk rotate y*A2}
cylinder{<0, C2.y, C1.z>, <0, C2.y, C2.z>, Thk rotate y*A1}
cylinder{<0, C2.y, C1.z>, <0, C2.y, C2.z>, Thk rotate y*A2}
sphere{<0, C1.y, C1.z>, Thk rotate y*A1}
sphere{<0, C2.y, C1.z>, Thk rotate y*A2}
sphere{<0, C1.y, C2.z>, Thk rotate y*A1}
sphere{<0, C2.y, C2.z>, Thk rotate y*A2}
sphere{<0, C1.y, C1.z>, Thk rotate y*A2}
sphere{<0, C2.y, C1.z>, Thk rotate y*A1}
sphere{<0, C1.y, C2.z>, Thk rotate y*A2}
sphere{<0, C2.y, C2.z>, Thk rotate y*A1}
#end
// corner 1, corner 2, iterations (counting down)
#macro boxDivide(C1, C2, I)
#local Gap = .01;
#local Size = <abs(C2.x-C1.x), abs(C2.y-C1.y), abs(C2.z-C1.z)>;
#if(1)
WireBox_Wedge(C1, C2, Gap)
#else
WireBox(C1, C2, Gap)
#end
#local R = Lerp(.5, rand(Seed), .5);
#if(I>0)
#if(Size.x>=Size.y & Size.x>=Size.z) // x is greater than or equal to y &/ z
boxDivide(<Lerp(C1.x, C2.x, R), C1.y, C1.z>+Gap, C2-Gap, I-1)
boxDivide(C1+Gap, <Lerp(C1.x, C2.x, R), C2.y, C2.z>-Gap, I-1)
#else
#if(Size.y>=Size.z) // y is greater than or equal to z
boxDivide(<C1.x, Lerp(C1.y, C2.y, R), C1.z>+Gap, C2-Gap, I-1)
boxDivide(C1+Gap, <C2.x, Lerp(C1.y, C2.y, R), C2.z>-Gap, I-1)
#else // z
boxDivide(<C1.x, C1.y, Lerp(C1.z, C2.z, R)>+Gap, C2-Gap, I-1)
boxDivide(C1+Gap, <C2.x, C2.y, Lerp(C1.z, C2.z, R)>-Gap, I-1)
#end
#end
#end
#end
union{
boxDivide(<-pi/3, -.5, .5>, <pi/3, .5, 1.5>, 7)
rotate -x*20
pigment{rgb .57}
normal{granite .1 scale .001}
}
Post a reply to this message
Attachments:
Download 'boxdivideb17m_43s.jpg' (93 KB)
Preview of image 'boxdivideb17m_43s.jpg'
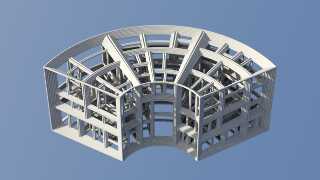
|
 |