|
 |
Okay, I've never posted to a newsgroup in my life, so if I mess it up,
forgive me. This is code for posable hands. They aren't perfect but kinda
fun to play with anyway. I've attach a GIF animation as well.
Janet
parrotdolphin.deviantart.com
// Persistence Of Vision raytracer version 3.6
#include "shapes.inc"
#include "metals.inc"
#include "golds.inc"
#include "colors.inc"
#include "textures.inc"
//#include "shapesq.inc"
//#include "rand.inc"
//#declare TEX = texture { Glass }
#declare TEX = texture { T_Gold_3C }
#declare TEX = texture { pigment { red 1 green .9 blue .75 }
finish { ambient .3 reflection .05 diffuse .6 } }
#declare NailColor = pigment { red .6 green 0 blue .1 }
//CAMERA
camera {location <0, 0, -24>
up< 0,1,0 >
right< 1.3333,0,0 >
look_at <0,0,0>}
//LIGHT
light_source {<0,7500,-7500> White }
//SKY
sky_sphere {
pigment {
agate
color_map {
// [ 0.7 color red 0 green 0 blue .2 ]
// [ 1.0 color red .35 green .35 blue .4 ]
[ 0.5 color red .55 green .5 blue .9 ]
[ 1.0 color red .9 green .8 blue 1.0 ]
}
turbulence .2
scale 1
rotate < 0, 0, 0 > }
}
// The following 5 variables can be altered to make the fingers longer or
shorter
#declare len1 = 3.5 ; //length of bottom joint
#declare len2 = 3 ; //length of middle joint
#declare len3 = 2.2 ; //length of top joint
#declare lent = 3.0 ; //length of thumb joints
#declare lenp = 3.5 ; //half the length of the palm
// The following variables can be altered to make the fingers bend and
spread
// Note - clock is for animations. colck* can be removed for still images.
// Looks like clock defaults to 0.
#declare aP = clock*5; //angle at which finger joints bend - PINKY
#declare saP = clock*15; //angle of finger spread - PINKY
#declare aR = clock*13; //angle at which finger joints bend - RING
#declare saR = clock*13; //angle of finger spread - RING
#declare aM = clock*21; //angle at which finger joints bend - MIDDLE
#declare saM = clock*11; //angle of finger spread - MIDDLE
#declare aI = clock*29; //angle at which finger joints bend - INDEX
#declare saI = clock*9; //angle of finger spread - INDEX
#declare aT = clock*32; //angle at which thumb joints bend - THUMB
#declare saT = 45; //angle of thumb spread - THUMB
#declare arP = (aP/180)*pi ; //angle in radians
#declare arR = (aR/180)*pi ; //angle in radians
#declare arM = (aM/180)*pi ; //angle in radians
#declare arI = (aI/180)*pi ; //angle in radians
#declare arT = (aT/180)*pi ; //angle in radians
//FINGER NAIL
#declare FingerNail =
difference {
sphere { <0,0,-2.25>, .5 scale y*2 scale z*.3 }
cone { len3*y, 0.6, -len3*y, 0.8 }
pigment { NailColor } finish { ambient .1 reflection .4 phong .3 }
}
//THUMBNAIL
#declare ThumbNail =
difference {
sphere { <0,0,-2.375>, .5 scale y*2 scale z*.3 }
cone { lenp*y, 0.6, -lenp*y, 0.8 }
pigment { NailColor } finish { ambient .1 reflection .4 phong .3 }
}
//FINGERS
#declare FINGER_P =
union{
union {
cone { 0*y, 0.9, -len1*y, 1 } //lower
sphere { <0,0,0>, 0.9 }
sphere { <0,-len1,0>, 1 }
rotate <aP,0,0>
translate < 0,len1*(cos(arP)),len1*(sin(arP)) >
}
union {
cone { 0*y, 0.8, -len2*y, 0.9 } //middle
sphere { <0,0,0>, 0.8 }
rotate <aP*2,0,0>
translate
<0,len1*cos(arP)+len2*cos(2*arP),len1*sin(arP)+len2*sin(2*arP)>
}
union {
cone { 0*y, 0.7, -len3*y, 0.8 } //upper
sphere { <0,0,0>, 0.7 }
object { FingerNail }
rotate <aP*3,0,0>
translate
<0,len1*cos(arP)+len2*cos(2*arP)+len3*cos(3*arP),len1*sin(arP)+len2*sin(2*arP)+len3*sin(3*arP)>
}
texture { TEX }
}
#declare FINGER_R = //inner 2 fingers
union{
union {
cone { 0*y, 0.9, -len1*y, 1 } //lower
sphere { <0,0,0>, 0.9 }
//sphere { <0,-len1,0>, 1 }
rotate <aR,0,0>
translate < 0,len1*(cos(arR)),len1*(sin(arR)) >
}
union {
cone { 0*y, 0.8, -len2*y, 0.9 } //middle
sphere { <0,0,0>, 0.8 }
rotate <aR*2,0,0>
translate
<0,len1*cos(arR)+len2*cos(2*arR),len1*sin(arR)+len2*sin(2*arR)>
}
union {
cone { 0*y, 0.7, -len3*y, 0.8 } //upper
sphere { <0,0,0>, 0.7 }
object { FingerNail }
rotate <aR*3,0,0>
translate
<0,len1*cos(arR)+len2*cos(2*arR)+len3*cos(3*arR),len1*sin(arR)+len2*sin(2*arR)+len3*sin(3*arR)>
}
texture { TEX }
}
#declare FINGER_M = //inner 2 fingers
union{
union {
cone { 0*y, 0.9, -len1*y, 1 } //lower
sphere { <0,0,0>, 0.9 }
//sphere { <0,-len1,0>, 1 }
rotate <aM,0,0>
translate < 0,len1*(cos(arM)),len1*(sin(arM)) >
}
union {
cone { 0*y, 0.8, -len2*y, 0.9 } //middle
sphere { <0,0,0>, 0.8 }
rotate <aM*2,0,0>
translate
<0,len1*cos(arM)+len2*cos(2*arM),len1*sin(arM)+len2*sin(2*arM)>
}
union {
cone { 0*y, 0.7, -len3*y, 0.8 } //upper
sphere { <0,0,0>, 0.7 }
object { FingerNail }
rotate <aM*3,0,0>
translate
<0,len1*cos(arM)+len2*cos(2*arM)+len3*cos(3*arM),len1*sin(arM)+len2*sin(2*arM)+len3*sin(3*arM)>
}
texture { TEX }
}
#declare FINGER_I =
union{
union {
cone { 0*y, 0.9, -len1*y, 1 } //lower
sphere { <0,0,0>, 0.9 }
sphere { <0,-len1,0>, 1 }
rotate <aI,0,0>
translate < 0,len1*(cos(arI)),len1*(sin(arI)) >
}
union {
cone { 0*y, 0.8, -len2*y, 0.9 } //middle
sphere { <0,0,0>, 0.8 }
rotate <aI*2,0,0>
translate
<0,len1*cos(arI)+len2*cos(2*arI),len1*sin(arI)+len2*sin(2*arI)>
}
union {
cone { 0*y, 0.7, -len3*y, 0.8 } //upper
sphere { <0,0,0>, 0.7 }
object { FingerNail }
rotate <aI*3,0,0>
translate
<0,len1*cos(arI)+len2*cos(2*arI)+len3*cos(3*arI),len1*sin(arI)+len2*sin(2*arI)+len3*sin(3*arI)>
}
texture { TEX }
}
//THUMB - LEFT
#declare Thumb_L =
union{
union {
cone { 0*y, 0.9, -lent*y, 1 } //lower
sphere { <0,0,0>, 0.9 }
sphere { <0,-lent,0>, 1 }
rotate <aT,0,0>
translate <0,lent*cos(arT),lent*sin(arT)>
}
union {
cone { 0*y, 0.8, -lent*y, 0.9 } //upper
sphere { <0,0,0>, 0.8 }
object { ThumbNail }
rotate <aT*2,0,0>
translate
<0,lent*cos(arT)+lent*cos(2*arT),lent*sin(arT)+lent*sin(2*arT)>
}
rotate y*-30
rotate z*-saT
translate <2.7,-lenp*1.25,0>
texture { TEX }
}
//THUMB - RIGHT
#declare Thumb_R =
union{
union {
cone { 0*y, 0.9, -lent*y, 1 } //lower
sphere { <0,0,0>, 0.9 }
sphere { <0,-lent,0>, 1 }
rotate <aT,0,0>
translate <0,lent*cos(arT),lent*sin(arT)>
}
union {
cone { 0*y, 0.8, -lent*y, 0.9 } //upper
sphere { <0,0,0>, 0.8 }
object { ThumbNail }
rotate <aT*2,0,0>
translate
<0,lent*cos(arT)+lent*cos(2*arT),lent*sin(arT)+lent*sin(2*arT)>
}
rotate y*30
rotate z*saT
translate <-2.7,-lenp*1.25,0>
texture { TEX }
}
//PALM
#declare Palm =
union{
cone { -2.7*x, .84, -.9*x, 1 } //across
cone { -.9*x, 1, .9*x, .88 } //across
cone { .9*x, .88, 2.4*x, .7 } //across
cone { 0*y, .84, -lenp*y, 1 //left top
translate < -2.7,0,0> }
cone { 0*y, .7, -lenp*y, 1 //right top
translate <2.4,0,0> }
cone { -lenp*y, 1, -lenp*2*y, 1 //left bottom
translate < -2.7,0,0> }
cone { -lenp*y, 1, -lenp*2*y, 1 //right bottom
translate <2.4,0,0> }
//triangles for back of hand top half
triangle { <-2.7,0,-.84>,<-2.7,-lenp,-1>,<-.9,0,-1> }
triangle { <-.9,0,-1>,<-2.7,-lenp,-1>,<0,-lenp,-1> }
triangle { <-.9,0,-1>,<0,-lenp,-1>,<.9,0,-.88> }
triangle { <0,-lenp,-1>,<.9,0,-.88>,<2.4,-lenp,-1> }
triangle { <.9,0,-.88>,<2.4,-lenp,-1>,<2.4,0,-.7> }
//triangles for back of hand bottom half
triangle { <-2.7,-lenp,-1>,<0,-lenp,-1>,<-2.7,-lenp*2,-1> }
triangle { <-2.7,-lenp*2,-1>,<0,-lenp,-1>,<2.4,-lenp*2,-1> }
triangle { <0,-lenp,-1>,<2.4,-lenp*2,-1>,<2.4,-lenp,-1> }
//triangles for palm of hand top half
triangle { <-2.7,0,.84>,<-2.7,-lenp,1>,<-.9,0,1> }
triangle { <-.9,0,1>,<-2.7,-lenp,1>,<0,-lenp,1> }
triangle { <-.9,0,1>,<0,-lenp,1>,<.9,0,.88> }
triangle { <0,-lenp,1>,<.9,0,.88>,<2.4,-lenp,1> }
triangle { <.9,0,.88>,<2.4,-lenp,1>,<2.4,0,.7> }
//triangles for palm of hand bottom half
triangle { <-2.7,-lenp,1>,<0,-lenp,1>,<-2.7,-lenp*2,1> }
triangle { <-2.7,-lenp*2,1>,<0,-lenp,1>,<2.4,-lenp*2,1> }
triangle { <0,-lenp,1>,<2.4,-lenp*2,1>,<2.4,-lenp,1> }
texture { TEX }
}
//RIGHTHAND
#declare RightHand =
union{
object { Palm }
object { Thumb_R }
object { FINGER_I scale .84 rotate <0,0,saI*1.5> translate < -2.7,0,0> }
object { FINGER_M scale 1 rotate <0,0,saM*.5> translate < -.9,0,0> }
object { FINGER_R scale .88 rotate <0,0,-saR*.5> translate <.9,0,0> }
object { FINGER_P scale .7 rotate <0,0,-saP*1.5> translate <2.4,0,0> }
}
//LEFTHAND
#declare LeftHand =
union{
object { Palm rotate y*180 }
object { Thumb_L }
object { FINGER_I scale .84 rotate <0,0,-saI*1.5> translate < 2.7,0,0> }
object { FINGER_M scale 1 rotate <0,0,-saM*.5> translate < .9,0,0> }
object { FINGER_R scale .88 rotate <0,0,saR*.5> translate <-.9,0,0> }
object { FINGER_P scale .7 rotate <0,0,saP*1.5> translate <-2.4,0,0> }
}
union {
object { LeftHand rotate <0,0,0> translate < -8,0,0>}
}
union {
object { RightHand rotate <0,0,0> translate < 8,0,0>}
}
Post a reply to this message
Attachments:
Download 'fingersanim2thumb.gif' (576 KB)
Preview of image 'fingersanim2thumb.gif'
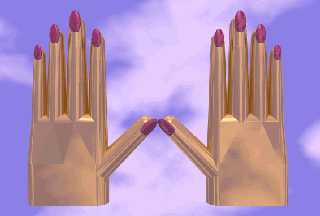
|
 |