 |
 |
|
 |
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
I ran some code which calculated some mathematically correct spirals and set
a scoring system based on the following criteria:
There are a reasonable number of iterations
The rotation of the outer square is close to 90 degrees
The size of the outer square is close to 5x5
Any two of these conditions can be met easily, but it looks like this is
about as close as you can get to satisfying all three while maintaining
mathematically correct placement.
In this image there are 47 squares, the final square is rotated by 89.994
degrees and the length of its side is 4.564
I've posted a PNG image because any JPG that didn't have absolutely awful
compression artefacts was considerably larger than this PNG.
#include "colors.inc"
#include "textures.inc"
#include "metals.inc"
#version 3.5;
global_settings {
assumed_gamma 2.2
max_trace_level 25
}
light_source {< 500, 500, -500> White * 1.0}
background {White}
camera {orthographic
location <0,0,-7>
look_at <0,0,3>
}
#declare WallTex = texture {pigment {Red} finish {ambient 0.5}}
#declare X = 1;
#declare Z = 0;
#declare N = 0;
#declare WallFan =
union {
#while (Z <= 90)
difference {
box {<-X,-X,-0.01>,<X,X,0.01> texture {WallTex}}
box {<-X+0.05,-X+0.05,-0.02>,<X-0.05,X-0.05,0.02> texture
{WallTex}}
rotate <0,0,Z>
}
// Length of new hypotenuse
#declare H = X * 1.032 + 0.003;
// solve simulataneous equations A+B=H, A^2 + B^2 = X^2
#declare A = (2*H + sqrt(4*H*H - 8*(H*H - X*X)))/4;
// use trig to find the angle
#declare Z = Z + degrees(acos(A/X));
#declare X = H;
#end
}
object {WallFan}
// Size reference box behind wall fan
box {<-5,-5,-0.005>,<5,5,0.005> pigment {Blue}}
Post a reply to this message
Attachments:
Download 'WallFan.png' (129 KB)
Preview of image 'WallFan.png'
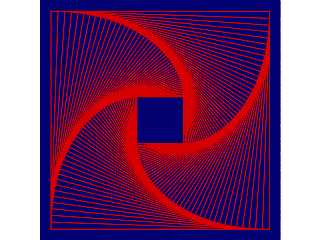
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
That code is amazing. I will probably scale other items to match the 4.564
size it ended up with. Very nice and WAY over my head!
"Mike Williams" <mik### [at] econym demon co uk> wrote in message
news:3f84fc53@news.povray.org...
> I ran some code which calculated some mathematically correct spirals and
set
> a scoring system based on the following criteria:
>
> There are a reasonable number of iterations
> The rotation of the outer square is close to 90 degrees
> The size of the outer square is close to 5x5
>
> Any two of these conditions can be met easily, but it looks like this is
> about as close as you can get to satisfying all three while maintaining
> mathematically correct placement.
>
> In this image there are 47 squares, the final square is rotated by 89.994
> degrees and the length of its side is 4.564
>
> I've posted a PNG image because any JPG that didn't have absolutely awful
> compression artefacts was considerably larger than this PNG.
>
> #include "colors.inc"
> #include "textures.inc"
> #include "metals.inc"
>
> #version 3.5;
> global_settings {
> assumed_gamma 2.2
> max_trace_level 25
> }
>
> light_source {< 500, 500, -500> White * 1.0}
>
> background {White}
>
> camera {orthographic
> location <0,0,-7>
> look_at <0,0,3>
> }
>
> #declare WallTex = texture {pigment {Red} finish {ambient 0.5}}
>
> #declare X = 1;
> #declare Z = 0;
> #declare N = 0;
> #declare WallFan =
> union {
> #while (Z <= 90)
> difference {
> box {<-X,-X,-0.01>,<X,X,0.01> texture {WallTex}}
> box {<-X+0.05,-X+0.05,-0.02>,<X-0.05,X-0.05,0.02> texture
> {WallTex}}
> rotate <0,0,Z>
> }
> // Length of new hypotenuse
> #declare H = X * 1.032 + 0.003;
> // solve simulataneous equations A+B=H, A^2 + B^2 = X^2
> #declare A = (2*H + sqrt(4*H*H - 8*(H*H - X*X)))/4;
> // use trig to find the angle
> #declare Z = Z + degrees(acos(A/X));
> #declare X = H;
> #end
> }
>
> object {WallFan}
>
> // Size reference box behind wall fan
> box {<-5,-5,-0.005>,<5,5,0.005> pigment {Blue}}
>
>
>
>
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
Awww, I'm jealous of Mike. I solved this problem a couple of years ago,
but I found the thread too late to be the first to post it. You might
still enjoy my code, as it is fairly efficient, well commented, and it
is an animation as well! Try it out, as it looks pretty cool. For the
still I have included, the clock value was .05. I enjoyed working out
this problem. It required a similar trigonometric solution as the ones
presented above. Now that I look at the equation involved, I wonder how
I got it. Oh well.
-Ben
//Title: Equiangular Spiral Animation
//Author: Ben Scheele
//Date: 5-20-2001
//updated 10-9-03
//animation .ini settings
//[300X300 AA on]
//+w300 +h300 +ki0 +kf1 +kfi1 +kff90 +A0.3 -j
camera{
location 20*y
up y right x
look_at 0
angle 6
}
light_source{ <20,50,20> rgb 1.25 }
background{ rgb .2}
#declare s = 1;
#declare a = 1;
#declare n = .06; //determines thickness of the frame
#declare T = 0;
#declare EndT = 90; //how far the square is rotated; sets the number of
objects as well
//set at 360 for the animation
#declare Step = 0+90*clock; //determines rotation rate
#while(T < EndT + Step )
#declare a = a/(sin(Step/180*pi)+cos(Step/180*pi))*.97; //all the
trig that's involved. (the .97 just shrinks it a bit)
difference{
box{ <-s,0,-s>, <s,s/4,s>}
box{ <-s+n,-.01,-s+n>, <s-n,s/4+.01,s-n> }
pigment{ rgb <a,.25,1-a> } //changes as it goes through the loop
finish{ ambient .2 diffuse .8 phong .4 reflection{ .2 .5}
metallic .3 specular.2 roughness .02 }
scale <a,1+5*T/EndT,a> // you can scale it more or less in the
vertical direction (y)
rotate T*y
}
#declare T = T + Step;
#end
Post a reply to this message
Attachments:
Download 'equiangular spiral.jpg' (36 KB)
Preview of image 'equiangular spiral.jpg'
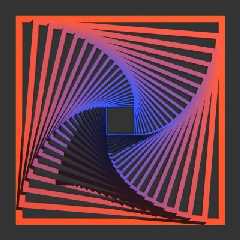
|
 |
|  |
|  |
|
 |
|
 |
|  |
|  |
|
 |
oops, sorry about the wrapping of the comments.
Post a reply to this message
|
 |
|  |
|  |
|
 |
|
 |
|  |
|
 |