|
 |
"GioSeregni" <gms### [at] hotmail com> wrote:
> First of all, I apologize for the naivety of the question.
> I am not able to get the surfaces of the BOX with the actual texture.
>
> Example:
>
> box{<0.5000,1.0000,0.2500>,<-3,-1.0000,-0.2500>
> texture {pigment {image_map
> {png "130PINK" map_type 1
> interpolate 2
> }
> scale 1.30
> }}
> rotate<0,360.0000,0> translate<46.7000,-0.0900,14.0700>
> }
>
> Well, Map 0 fails on some faces, Map 1 and map 2 deform the texture.
> How can I wrap a texture correctly?
> I studied the UV Mapping, but I practically understood nothing.
> How should I correct my example to get the same texture uniformly on all faces?
> A huge thank you if anyone helps me.
>
> BR
> Giovanni
There is no 'box' mapping in POV. You could hand code the box out of clipped
planes, mapping each side by rotating map 'type 0' to face each side. There's
probably a macro that someone has written to do just that.
I wrote a macro that 'box maps' the Round_Box macro. It can simulate a square
edged box if you set the edge radius fillet very low.
Miller
// make mapped box
// diced Round_Box() into a 6 sided uv mapped Round Box
// m.miller 05.21.23
#include "shapes.inc"
#include "shapes2.inc"
#macro make_mapped_box (w,h,d,f,mat,z_dir)
#declare _form = object{ Round_Box(<-w/2,0,0>,<w/2,h,d>, f , 1) }
union {
// X right
difference {
object { _form }
plane { <1,0,0>, 0 translate <w/2-f/2,0,0> }
plane { <1,0,0>, 0 rotate <0,-45,0> translate <w/2,0,0> }
plane { <1,0,0>, 0 rotate <0,45,0> translate <w/2,0,d> }
plane { <0,-1,0>, 0 rotate <0,0,45> translate <w/2,h,0> }
plane { <0, 1,0>, 0 rotate <0,0,-45> translate <w/2,0,0> }
material {mat rotate <0,-90,0> translate <0,0,0> }
#if (z_dir)
material {mat rotate <0,-90,0> rotate <90,0,0> translate <0,0,0> }
#end
rotate <0,0,0>
translate <0,0,0>
}
// X left
difference {
object { _form }
plane { <1,0,0>, 0 translate <w/2-f/2,0,0> }
plane { <1,0,0>, 0 rotate <0,-45,0> translate <w/2,0,0> }
plane { <1,0,0>, 0 rotate <0,45,0> translate <w/2,0,d> }
plane { <0,-1,0>, 0 rotate <0,0,45> translate <w/2,h,0> }
plane { <0, 1,0>, 0 rotate <0,0,-45> translate <w/2,0,0> }
material {mat rotate <0,-90,0> translate <0,0,0> }
#if (z_dir)
material {mat rotate <0,-90,0> rotate <90,0,0> translate <0,0,0> }
#end
rotate <0,180,0>
translate <0,0,d>
}
// Y top/bottom
difference {
object { _form }
plane {<0,-1,0> 0 rotate <0,0,0> translate <0,f/2,0>}
plane {<0,-1,0> 0 rotate <-45,0,0> translate <0,0,0>}
plane {<0,-1,0> 0 rotate <45,0,0> translate <0,0,d>}
plane {<0,-1,0> 0 rotate <0,0,-45> translate <w/2,0,0>}
plane {<0,-1,0> 0 rotate <0,0,45> translate <-w/2,0,0>}
material {mat rotate <-90,180,0> translate <-w/2,0,0> }
rotate <0,0,0>
translate <0,0,0>
}
// Y top/bottom
difference {
object { _form }
plane {<0,-1,0> 0 rotate <0,0,0> translate <0,f/2,0>}
plane {<0,-1,0> 0 rotate <-45,0,0> translate <0,0,0>}
plane {<0,-1,0> 0 rotate <45,0,0> translate <0,0,d>}
plane {<0,-1,0> 0 rotate <0,0,-45> translate <w/2,0,0>}
plane {<0,-1,0> 0 rotate <0,0,45> translate <-w/2,0,0>}
material {mat rotate <-90,180,0> translate <-w/2,0,0> }
rotate <0,0,180>
translate <0,h,0>
}
// Y front
difference {
object { _form }
plane { <0,0,-1>, 0 translate <0,0,f/2> }
plane {<-1,0,0>,0 rotate <0,-45,0> translate <w/2,0,0> }
plane {<1,0,0>,0 rotate <0,45,0> translate <-w/2,0,0> }
plane {<0,-1,0>,0 rotate <45,0,0> translate <0,h,0> }
plane {<0,1,0>,0 rotate <-45,0,0> translate <0,0,0> }
material {mat rotate <0,0,0> translate <-w/2,0,0> }
rotate <0,0,0>
translate <0,0,0>
}
// Y back
difference {
object { _form }
plane { <0,0,-1>, 0 translate <0,0,f/2> }
plane {<-1,0,0>,0 rotate <0,-45,0> translate <w/2,0,0> }
plane {<1,0,0>,0 rotate <0,45,0> translate <-w/2,0,0> }
plane {<0,-1,0>,0 rotate <45,0,0> translate <0,h,0> }
plane {<0,1,0>,0 rotate <-45,0,0> translate <0,0,0> }
material {mat rotate <0,0,0> translate <-w/2,0,0> }
rotate <0,180,0>
translate <0,0,d>
}
}
#end
---------------------------------------
Usage example:
#declare _mat = M_wood;
#declare _z_dir = 1;
#declare _fillet = .2;
#declare _width = 5;
#declare _height = 5;
#declare _depth = 10;
object { make_mapped_box(_width, _height, _depth, _fillet, _mat, _z_dir)
translate <0,0,-_depth/2> rotate <0,30,0> translate <-10,0,0>}
Post a reply to this message
Attachments:
Download 'box_mapped_uv.png' (703 KB)
Preview of image 'box_mapped_uv.png'
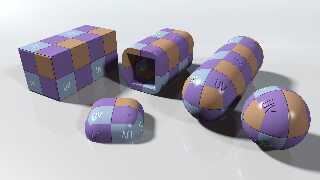
|
 |