|
 |
"Bald Eagle" <cre### [at] netscape net> wrote:
> So, I have read about the octree algorithm, and I can follow along and make
> sense of what's going on, however -
> (and maybe I need to go through it in a much more detailed level)
> One of the interesting things about an octree is "saving" the leaves and
> rejecting the empty voxels so that later you can quickly traverse the octree.
> Maybe that's what he had developed later, before the HDD crash, but I think
> that's a tool that a lot of Povvers could use, and I'm interested in learning
> about that aspect of it, and coding it up as time permits.
>
> Also, I don't see that Robert used a difference{}, and so I'm unsure how you're
> suggesting I implement that second object and where it gets referenced.
Here is a test scene to play with.
#version 3.7;
#declare RAD = 4;
global_settings {
#declare a_g = 2.2;
assumed_gamma a_g
max_trace_level 5
noise_generator 2
#if(RAD > 0)
radiosity {
pretrace_start 0.08
pretrace_end 0.04/RAD
count 30*RAD
nearest_count min (20, RAD)
error_bound 2/RAD
low_error_factor 0.5
recursion_limit 1
gray_threshold 0
minimum_reuse 0.015
brightness 1
adc_bailout 0.005
normal on
media on
}
#end
}
#include "stdinc.inc"
sky_sphere {
pigment {
average
pigment_map {
[1 function {max (min (y, 1), 0)}
color_map {
[0 rgb 2.35]
[0.96 rgb 4.5]
[1 rgb 13.5]
}
]
[1 function {max (min (y, 1), 0)}
turbulence 0.2
color_map {
[0 rgbt 1]
[0.5 rgbt <0.385,0.77,1.1,1>]
}
rotate z*-45
]
}
}
}
camera {
location <2,2,-4>
look_at <0.1,0,0>
right x*image_width/image_height
up y
angle 82
}
light_source {
<-80000,50000,-50000>,
color rgb <2.42,2.23,1.87>
}
plane {y, -1.6 finish {reflection {0, 1}}}
//______________________________________________________________________________
#declare obj_ =
isosurface {
function {f_sphere (x,y,z,1)-f_noise3d (x*2.5,y*2.5,z*2.5)*0.6}
contained_by {sphere {0, 1.6}}
max_gradient 2.5
hollow
texture {
pigment {color rgb <0.35,0.32,0.55>}
finish {reflection {0, 1}}
}
}
object {obj_}
#declare rootObj =
difference {
object {obj_ scale <2.5, 1.4,1.4>}
object {obj_}
}
#declare thin = 0.02;
#declare frame =
union {
cylinder {<-1,-1,-1>, <-1,1,-1>, thin}
cylinder {<-1,-1,1>, <-1,1,1>, thin}
cylinder {<1,-1,-1>, <1,1,-1>, thin}
cylinder {<1,-1,1>, <1,1,1>, thin}
cylinder {<-1,-1,-1>, <1,-1,-1>, thin}
cylinder {<1,-1,-1>, <1,-1,1>, thin}
cylinder {<1,-1,1>, <-1,-1,1>, thin}
cylinder {<-1,-1,1>, <-1,-1,-1>, thin}
cylinder {<-1,1,-1>, <1,1,-1>, thin}
cylinder {<1,1,-1>, <1,1,1>, thin}
cylinder {<1,1,1>, <-1,1,1>, thin}
cylinder {<-1,1,1>, <-1,1,-1>, thin}
sphere {<-1,-1,-1>, thin}
sphere {<1,-1,-1>, thin}
sphere {<-1,1,-1>, thin}
sphere {<-1,-1,1>, thin}
sphere {<-1,1,1>, thin}
sphere {<1,-1,1>, thin}
sphere {<1,1,-1>, thin}
sphere {<1,1,1>, thin}
}
#declare MAX_DEPTH = 8;
#declare R = seed (0);
#declare OUTSIDE = 0;
#declare PARTIAL = 1;
#declare INSIDE = 2;
#declare _nDepth = 0;
#declare _nBoxes = 0;
#declare _nNullBoxes = 0;
#macro GAUSS (X)
((rand (X)+rand (X)+rand (X)+rand (X)+rand (X)+rand (X)-3)*1)
#end
#macro GetBoxState (obj_, bbox)
#local bmin = min_extent (bbox);
#local bmax = max_extent (bbox);
#local n = 0;
#if (inside (obj_, <bmin.x,bmin.y,bmin.z>)) #local n = n+1; #end
#if (inside (obj_, <bmax.x,bmax.y,bmax.z>)) #local n = n+1; #end
#if (inside (obj_, <bmin.x,bmax.y,bmin.z>)) #local n = n+1; #end
#if (inside (obj_, <bmax.x,bmax.y,bmin.z>)) #local n = n+1; #end
#if (inside (obj_, <bmax.x,bmin.y,bmin.z>)) #local n = n+1; #end
#if (inside (obj_, <bmin.x,bmin.y,bmax.z>)) #local n = n+1; #end
#if (inside (obj_, <bmin.x,bmax.y,bmax.z>)) #local n = n+1; #end
#if (inside (obj_, <bmax.x,bmin.y,bmax.z>)) #local n = n+1; #end
#switch (n)
#case (0)
#local state = OUTSIDE;
#break
#case (8)
#local state = INSIDE;
#break
#else
#local state = PARTIAL;
#break
#end
state
#end
#macro BuildDisplayVoxel (bbox)
#local clr1 = srgb <225,180,105>/255;
#local clr2 = srgb <105,90,30>/255;
#local gry = RRand (0.1, 0.9, R);
#local bmin = min_extent (bbox);
#local bmax = max_extent (bbox);
#local ctr = (bmin+bmax)*0.5;
union {
object {frame}
rotate y*90
scale _rootScale
pigment {color rgb <1,0,0>}
scale vlength (ctr-bmax)
translate ctr
}
#declare _nBoxes = _nBoxes+1;
#end
#macro SubdivideVoxel (root, bbParent)
#declare _nDepth = _nDepth+1;
#if (_nDepth <= MAX_DEPTH) // break into 8 sub-boxes
#local aBox = array [8];
#local bmin = min_extent (bbParent);
#local bmax = max_extent (bbParent);
#local midX = (bmin.x+bmax.x)*0.5;
#local midY = (bmin.y+bmax.y)*0.5;
#local midZ = (bmin.z+bmax.z)*0.5;
#local aBox [0] = box {<bmin.x,bmin.y,bmin.z>,<midX, midY, midZ>}
#local aBox [1] = box {<bmin.x,midY, bmin.z>,<midX, bmax.y,midZ>}
#local aBox [2] = box {<midX, bmin.y,bmin.z>,<bmax.x,midY, midZ>}
#local aBox [3] = box {<midX, midY, bmin.z>,<bmax.x,bmax.y,midZ>}
#local aBox [4] = box {<bmin.x,bmin.y,midZ>, <midX, midY, bmax.z>}
#local aBox [5] = box {<bmin.x,midY, midZ>, <midX, bmax.y,bmax.z>}
#local aBox [6] = box {<midX, bmin.y,midZ>, <bmax.x,midY, bmax.z>}
#local aBox [7] = box {<midX, midY, midZ>, <bmax.x,bmax.y,bmax.z>}
#local n = 0;
#while (n < 8)
#if (INSIDE = GetBoxState (root, aBox [n]))
BuildDisplayVoxel (aBox [n])
#else
#if (PARTIAL = GetBoxState (root, aBox [n]))
SubdivideVoxel (root, aBox [n])
#else
#declare _nNullBoxes = _nNullBoxes+1;
#local isect =
intersection {
object {root}
object {aBox [n]}
}
#local bmin = min_extent (isect);
#if (bmin.x > -10000000000)
SubdivideVoxel (root, aBox [n])
#end
#end
#end
#local n = n+1;
#end
#end
#declare _nDepth = _nDepth-1;
#end
#macro SubdivideVoxelMain (root, bbParent)
#local aBox = array [8];
#local bmin = min_extent (bbParent);
#local bmax = max_extent (bbParent);
#local midX = (bmin.x+bmax.x)*0.5;
#local midY = (bmin.y+bmax.y)*0.5;
#local midZ = (bmin.z+bmax.z)*0.5;
#local aBox [0] = box {<bmin.x,bmin.y,bmin.z>,<midX, midY, midZ>}
#local aBox [1] = box {<bmin.x,midY, bmin.z>,<midX, bmax.y,midZ>}
#local aBox [2] = box {<midX, bmin.y,bmin.z>,<bmax.x,midY, midZ>}
#local aBox [3] = box {<midX, midY, bmin.z>,<bmax.x,bmax.y,midZ>}
#local aBox [4] = box {<bmin.x,bmin.y,midZ>, <midX, midY, bmax.z>}
#local aBox [5] = box {<bmin.x,midY, midZ>, <midX, bmax.y,bmax.z>}
#local aBox [6] = box {<midX, bmin.y,midZ>, <bmax.x,midY, bmax.z>}
#local aBox [7] = box {<midX, midY, midZ>, <bmax.x,bmax.y,bmax.z>}
#local n = 0;
#while (n < 8)
#if (INSIDE = GetBoxState (root, aBox [n]))
BuildDisplayVoxel (aBox [n])
#else
#if (PARTIAL = GetBoxState (root, aBox [n]))
SubdivideVoxel (root, aBox [n])
#else
SubdivideVoxel (root, aBox [n])
#end
#end
#local n = n+1;
#end
#end
#declare bmin = <-40,-40,-40>; //min_extent (rootObj);
#declare bmax = <40,40,40>; //max_extent (rootObj);
#declare ctr = (bmin+bmax)*0.5;
#declare bbRoot =
box {
vtransform (ctr, transform {translate -bmax}),
vtransform (ctr, transform {translate bmax})
}
#declare _rootScale = 20/vlength (ctr-bmax)*2;
union {
SubdivideVoxelMain (rootObj, bbRoot)
}
// end of code
Norbert
Post a reply to this message
Attachments:
Download 'octree_test_scene.jpg' (614 KB)
Preview of image 'octree_test_scene.jpg'
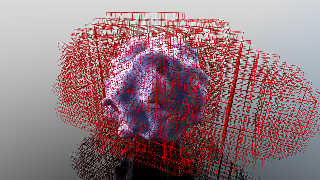
|
 |