|
 |
Le 01/01/2016 14:21, Le_Forgeron a écrit :
> For kurtz le pirarte, from povray.general
>
For
complete code :
// ---------------------------------------------------------------------
// ---------------------------------------------------------------------
// --- INCLUDES
// ---------------------------------------------------------------------
#include "colors.inc"
#include "myMacros.inc"
#declare displayAxis = false;
#default {
finish { ambient 0.40 diffuse 0.60 }
}
// ---------------------------------------------------------------------
// --- CURVE FUNCTION
//
// x = cos(t) + 2.cos(2.t)
// y = sin(t) - 2.sin(2.t)
// z = 2.e.sin(3.t)
// ---------------------------------------------------------------------
#macro myFunc(thisTime)
< cos(thisTime) + 2*cos(2*thisTime),
sin(thisTime)-2*sin(2*thisTime),
2*sin(3*thisTime)>
#end
// ---------------------------------------------------------------------
// --- MACROS
// ---------------------------------------------------------------------
// --- Returns a vector perpendicular to V1 and V2
#macro VPerp_To_Plane(V1, V2)
(vnormalize(vcross(V1, V2)))
#end
// -- VRotation() will find the rotation angle from V1 to V2 around Axis.
#macro VRotation(V1, V2, Axis)
(acos(min(vdot(vnormalize(V1),vnormalize(V2)),1))*(vdot(Axis,vcross(V1,V2))<0?-1:1))
#end
// -- Projects a vector onto the plane defined by Axis.
#macro VProject_Plane(V, Axis)
#local A = vnormalize(Axis);
(V - vdot(V, A)*A)
#end
#macro Matrix_Trans(A, B, C, D)
transform {
matrix < A.x, A.y, A.z,
B.x, B.y, B.z,
C.x, C.y, C.z,
D.x, D.y, D.z>
}
#end
#macro curve_Trans (Time, Sky, Foresight)
#local Location = <0,0,0> + myFunc(Time);
#local LocationNext = <0,0,0> + myFunc(Time + Foresight);
#local LocationPrev = <0,0,0> + myFunc(Time-Foresight);
#local Forward = vnormalize(LocationNext-Location);
#local Right = VPerp_To_Plane(Sky,Forward);
#local Up = VPerp_To_Plane(Forward,Right);
#local Matrix = Matrix_Trans(Right,Up,Forward,Location)
transform {
transform Matrix
}
#end
// ---------------------------------------------------------------------
// --- SCENE
// ---------------------------------------------------------------------
global_settings {
assumed_gamma 1.80
max_trace_level 10
}
camera {
location p2r(50, 25, 15,false)
// up y
// right x*image_width/image_height
look_at <0, 0, 0>
angle 36
}
light_source {
p2r(45, 45, 1000,false)
color White
}
background {
color White*0
}
// ---------------------------------------------------------------------
// --- GRID
// ---------------------------------------------------------------------
#if (displayAxis)
#declare gridSTep=0.50;
#declare axisLen = 9;
#declare gridColor = White*0.25;
drawAxis (axisLen + 0.50, 0.02)
#declare index=-axisLen*0.50;
#while (index<= + axisLen*0.50)
cylinder { <index, 0, -axisLen*0.50>, <index, 0, + axisLen*0.50>, 0.01
pigment { color gridColor } }
#declare index=index + gridSTep;
#end
#declare index = -axisLen*0.50;
#while (index<= + axisLen*0.50)
cylinder { <-axisLen*0.50, 0, index>, < + axisLen*0.50, 0, index>,
0.01 pigment { color gridColor } }
#declare index=index + gridSTep;
#end
#end
// ---------------------------------------------------------------------
// --- CURVE
// ---------------------------------------------------------------------
//
// x = cos(t) + 2.cos(2.t)
// y = sin(t) - 2.sin(2.t)
// z = 2.e.sin(3.t)
//
// e = + 1 pour droit (dextre)
// e = -1 pour gauche (senestre)
//
//
--------------------------------------------------------------------------
#declare deuxpi = 2*pi;
#declare r = 0.05;
#declare courbeFinish = finish {
phong 0.85
brilliance 2
};
#declare currentPt = <0.00, 0.00, 0.00>;
#declare previousPt = <0.00, 0.00, 0.00>;
#declare theColor = rgb <1.00, 1.00, 1.00>*0.80;
#declare tt=0;
#declare step=2*pi/360;
#while (tt<deuxpi)
#declare currentPt = myFunc(tt);
sphere { currentPt, r pigment { theColor } finish { courbeFinish } }
#if(tt!=0)
cylinder { previousPt, currentPt, r pigment { theColor } finish {
courbeFinish } }
#end
#declare previousPt = currentPt;
#declare tt=tt + step;
#end
#declare a = 0.40;
#declare b = 0.05;
// ---------------------------------------------------------------------
#declare cercle = torus {
a, b
rotate 90*x
pigment { color Goldenrod }
finish { courbeFinish }
}
// ---------------------------------------------------------------------
#declare p0=< + a, + a, 0>;
#declare p1=<-a, + a, 0>;
#declare p2=<-a, -a, 0>;
#declare p3=< + a, -a, 0>;
#declare cadre = union {
sphere { p0, 0.05 }
sphere { p1, 0.05 }
sphere { p2, 0.05 }
sphere { p3, 0.05 }
cylinder { p0 ,p1, b }
cylinder { p1 ,p2, b }
cylinder { p2 ,p3, b }
cylinder { p3 ,p0, b }
pigment { color Brass }
finish { courbeFinish }
}
// ---------------------------------------------------------------------
#declare p0=<0, a, 0>;
#declare p1=< + a*cos(radians(30)), -a*sin(radians(30)), 0>;
#declare p2=<-a*cos(radians(30)), -a*sin(radians(30)), 0>;
#declare Triangle = union {
sphere { p0, 0.05 }
sphere { p1, 0.05 }
sphere { p2, 0.05 }
cylinder { p0 ,p1, b }
cylinder { p1 ,p2, b }
cylinder { p2 ,p0, b }
pigment { color Brass*0.85 }
// pigment { color Goldenrod*0.75 }
finish { courbeFinish }
}
// ---------------------------------------------------------------------
// --- DRAW OBJECTS
// ---------------------------------------------------------------------
// curve_Trans (Time, Sky, Foresight)
#declare index=0;
#while(index<360)
object {
Triangle
curve_Trans (radians(index), y, 0.10)
}
#declare index=index + 2;
#end
---
kurtz le pirate
compagnie de la banquise
Post a reply to this message
Attachments:
Download 'probleme.jpg' (88 KB)
Preview of image 'probleme.jpg'
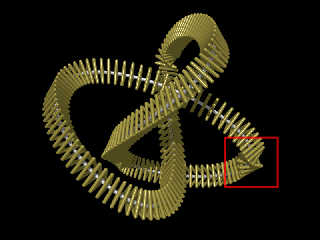
|
 |