|
 |
Here's an isosurface that I tried to do ages ago, & couldn't get it right,
because I didn't use pi properly with f_th() & f_ph(). Here's the code to
play with:
// ----------------------------------------
// Persistence of Vision Ray Tracer Scene Description File
// File: SpheriCoil.pov
// Vers: 3.6
// Desc: Spiral Ball, using Isosurface
// Date: 2005.09.10
// Auth: PM 2Ring
//
// -D +A0.1 +AM2 +R2
// -F +A0.6 +AM2 +R2
//
#version 3.6;
#include "math.inc"
#declare Do_Iso=1; //1: do isosurface object(s), 0: do
sphere placeholders
#declare Do_Single=0; //1: do a single object, 0: do a grid of
objects
#declare Do_Floor=1;
#declare Do_Sky=1;
global_settings {
assumed_gamma 1.0
//max_trace_level 10
}
// ----------------------------------------
//Centered modulus
#declare scmod = function(a,b) {mod(abs(a),2*b)-b}
//Spiral ball. Parameters. W: winding number, M: number of arms, CR:
Cylinder radius as a ratio
#macro SpheriCoil(W, M, CR)
#local CRad = CR / W; //Cylinder radius
#local BRad = 1 + 2*CRad; //Bounding radius
#local TAN = 0.5 * M / W ; //Tangent of angle that cylinders make
with X-axis
#local C = 1 / f_r(0, 1, TAN); //Cos
#local S = C * TAN; //Sin
#local A = pi * S / M; //Distance between cylinder centres
//Shape function
//cylinders parallel to x axis in xz plane
#local f_Cyls = function {f_r(0, y, scmod(z,A)) - CRad}
//rotate around y
#local f_Spy = function{f_Cyls(0, y, C*z-S*x)}
//wrap around sphere to get final function
#local f_SpyBall = function{f_Spy(f_th(x,y,z), 1-f_r(x,y,z), f_ph(x,y,z))}
//SpheriCoil
#if(Do_Iso)
isosurface{
function{f_SpyBall(x,y,z)}
contained_by {sphere{0, BRad}}
max_gradient 40
accuracy 5e-3
#else
sphere{0, BRad
#end
scale 1/BRad
texture{TRainbow}
//rotate 45*y
rotate -45*x
}
#end
// ---Textures-----------------------------
#declare cmRainbow =
color_map{
[0 red 1]
[0.166 rgb<1,1,0>]
[0.333 green 1]
[0.5 rgb<0,1,1>]
[0.666 blue 1]
[0.833 rgb<1,0,1>]
[1 red 1]
}
#declare TRainbow =
texture{
pigment{
radial
//onion scale .3 phase .75
color_map{cmRainbow}
}
finish{
specular .5 roughness 1e-3
ambient .1 diffuse .8 reflection .1
}
}
//---The Scene-------------------------------------
//Object(s)
#if(Do_Single)
//A single Sphericoil
#declare D = 3;
#declare MX = 1;
object{
SpheriCoil(3, 6, .5)
translate <0,1,1>
}
#else
//Make a grid of SpheriCoils
#declare D = 3;
#declare MX = 5;
#declare MZ = MX;
#declare Z=0;
#while(Z<MZ)
#declare X=0;
#while(X<MX)
object{
SpheriCoil(Z+1, X+1, .5)
translate <(X+.5-MX/2)*D, 1, (Z+.5-MZ/2)*D*1.5>
}
#declare X=X+1;
#if(X=0)#declare X = 1;#end
#end
#declare Z=Z+1;
#end
#end
//Stage
#if(Do_Sky)
sphere {0,1 inverse
pigment {
function{abs(y)}
color_map {
[0.0 rgb <.1, .15, .5>]
[0.6 rgb <.8,.8,1>]
[.8 rgb 1]
[1 rgb <1,1,.9>]
}
}
finish{ambient 1 diffuse 0} //No good for radiosity!!!
scale 125
}
#else
background{rgb .5}
#end
#if(Do_Floor)
plane {
y, -1e-3
pigment {
checker rgb .85, rgb 0 scale 1.5
rotate 45*y
}
finish{
ambient 0.1 diffuse 0.85
reflection 0.175
}
}
#end
// ----------------------------------------
camera {
right x*image_width/image_height up y
direction z
location <0, 3.75, -6> * MX * D * .28
look_at -y*.5
angle 40
}
light_source {<1,1,-2>*10 rgb 1}
// ----------------------------------------
Post a reply to this message
Attachments:
Download 'sphericoilbw0.jpg' (112 KB)
Preview of image 'sphericoilbw0.jpg'
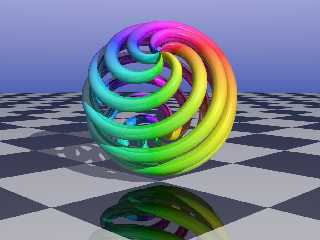
|
 |